ClimateDISK: Hockey stick#
This notebook attempts to follow the WINGS steps necessary to reproduce the hockey stick figure (and allow for upgrades as new data becomes available).
Let’s first import our favorite packages:
import json
import requests
import pandas as pd
import io
import ast
import numpy as np
import pyleoclim as pyleo
import seaborn as sns
import cfr as cfr
Step 1: Data query#
For now let’s query the LiPDverse v3 graph directly. For ClimateDisk, we may need to return the datasets URL, send the datasets to WINGS and use PyLiPD in the first component to produce the DataFrame.
url = 'https://linkedearth.graphdb.mint.isi.edu/repositories/LiPDVerse3'
query = """PREFIX le: <http://linked.earth/ontology#>
PREFIX wgs84: <http://www.w3.org/2003/01/geo/wgs84_pos#>
SELECT ?dataSetName ?archiveType ?geo_meanLat ?geo_meanLon ?geo_meanElev
?paleoData_variableName ?paleoData_values ?paleoData_units
?paleoData_proxy ?paleoData_proxyGeneral ?time_variableName ?time_values
?time_units ?compilationName ?TSID ?QAlabel where{
?ds a le:Dataset .
?ds le:name ?dataSetName .
OPTIONAL{?ds le:proxyArchiveType ?archiveType .}
?ds le:collectedFrom ?loc .
?loc wgs84:lat ?geo_meanLat .
?loc wgs84:long ?geo_meanLon .
OPTIONAL {?loc wgs84:alt ?geo_meanElev .}
?ds le:includesPaleoData ?data .
?data le:foundInMeasurementTable ?table .
?table le:includesVariable ?var .
?var le:name ?paleoData_variableName .
?var le:hasValues ?paleoData_values .
OPTIONAL{?var le:hasUnits ?paleoData_units .}
OPTIONAL{?var le:proxy ?paleoData_proxy .}
OPTIONAL{?var le:proxyGeneral ?paleoData_proxyGeneral .}
?var le:partOfCompilation ?compilation .
?compilation le:name ?compilationName .
VALUES ?compilationName {"iso2k" "Pages2kTemperature"} .
?var le:useInGlobalTemperatureAnalysis ?QAlabel .
FILTER (?QAlabel = True || regex(?QAlabel, "True.*", "i")) .
OPTIONAL{?var le:hasVariableID ?TSID} .
?table le:includesVariable ?timevar .
?timevar le:name ?time_variableName .
FILTER (regex(?time_variableName, "year.*")).
?timevar le:hasValues ?time_values .
OPTIONAL{?timevar le:hasUnits ?time_units .}
}"""
response = requests.post(url, data = {'query': query})
data = io.StringIO(response.text)
df = pd.read_csv(data, sep=",")
df['paleoData_values']=df['paleoData_values'].apply(lambda row : json.loads(row) if isinstance(row, str) else row)
df['time_values']=df['time_values'].apply(lambda row : json.loads(row) if isinstance(row, str) else row)
df.head()
dataSetName | archiveType | geo_meanLat | geo_meanLon | geo_meanElev | paleoData_variableName | paleoData_values | paleoData_units | paleoData_proxy | paleoData_proxyGeneral | time_variableName | time_values | time_units | compilationName | TSID | QAlabel | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | LS16STCL | LakeSediment | 50.830 | -116.39 | 1126.0 | d18O | [-7.81, -5.91, -9.03, -5.35, -5.61, -5.98, -5.... | permil | d18O | NaN | year | [2009.0, 2008.3, 2007.8, 2007.4, 2007.0, 2006.... | AD | iso2k | LPD7dc5b9ba | True |
1 | CO00URMA | Coral | 0.933 | 173.00 | 6.0 | d18O | [-4.8011, -4.725, -4.6994, -4.86, -5.0886, -5.... | permil | d18O | NaN | year | [1994.5, 1994.33, 1994.17, 1994.0, 1993.83, 19... | AD | iso2k | Ocean2kHR_177 | True |
2 | CO00URMA | Coral | 0.933 | 173.00 | 6.0 | d18O | [-4.8011, -4.725, -4.6994, -4.86, -5.0886, -5.... | permil | d18O | NaN | year | [1994.5, 1994.33, 1994.17, 1994.0, 1993.83, 19... | AD | iso2k | Ocean2kHR_177 | True |
3 | CO00URMA | Coral | 0.933 | 173.00 | 6.0 | d18O | [-4.8011, -4.725, -4.6994, -4.86, -5.0886, -5.... | permil | d18O | NaN | year | [1994.5, 1994.33, 1994.17, 1994.0, 1993.83, 19... | AD | iso2k | Ocean2kHR_177 | True |
4 | CO05KUBE | Coral | 32.467 | -64.70 | -12.0 | d18O | [-4.15, -3.66, -3.69, -4.07, -3.95, -4.12, -3.... | permil | d18O | NaN | year | [1983.21, 1983.13, 1983.04, 1982.96, 1982.88, ... | AD | iso2k | Ocean2kHR_105 | True |
"The number of records is "+str(len(df.index))
'The number of records is 656'
df['QAlabel'].unique()
array([ True])
df.columns
Index(['dataSetName', 'archiveType', 'geo_meanLat', 'geo_meanLon',
'geo_meanElev', 'paleoData_variableName', 'paleoData_values',
'paleoData_units', 'paleoData_proxy', 'paleoData_proxyGeneral',
'time_variableName', 'time_values', 'time_units', 'compilationName',
'TSID', 'QAlabel'],
dtype='object')
Step 2: Remove duplicate rows#
To be considered a duplicate:
Have the same
TSID
Have the same
paleoData_variableName
Have the same
paleoData_values
Have the same
time_values
def is_duplicate(row1,row2):
if row1['TSID']==row2['TSID']:
if row1['paleoData_variableName']==row2['paleoData_variableName']:
if len(row1['paleoData_values']) != len(row2['paleoData_values']):
equal=False
else:
if np.allclose(row1['paleoData_values'], row2['paleoData_values'], atol=0.001)==True:
if len(row1['time_values']) != len(row2['time_values']):
equal=False
else:
if np.allclose(row1['time_values'], row2['time_values'], atol=0.001)==True:
equal = True
else: equal=False
else:
equal=False
else:
equal=False
else:
equal = False
return equal
tsid = df['TSID'].unique()
idx_list = []
for item in tsid:
df1=df[df['TSID']==item]
if len(df1.index)>1:
comp = df1.iloc[0]
df2 = df1.iloc[1:]
for index, row in df2.iterrows():
if is_duplicate(comp,row)==True:
idx_list.append(index)
df_nodup = df.drop(idx_list)
"There is now "+ str(len(df_nodup.index)) + " timeseries available."
'There is now 612 timeseries available.'
df_nodup.columns
Index(['dataSetName', 'archiveType', 'geo_meanLat', 'geo_meanLon',
'geo_meanElev', 'paleoData_variableName', 'paleoData_values',
'paleoData_units', 'paleoData_proxy', 'paleoData_proxyGeneral',
'time_variableName', 'time_values', 'time_units', 'compilationName',
'TSID', 'QAlabel'],
dtype='object')
df_metadata = df_nodup.filter(['dataSetName','archiveType','geo_meanLat', 'geo_meanLon', 'geo_meanElev','paleoData_variableName','paleoData_units','paleoData_proxy','paleoData_proxyGeneral','time_variableName',' time_units','compilationName','TSID'])
df_metadata.columns
Index(['dataSetName', 'archiveType', 'geo_meanLat', 'geo_meanLon',
'geo_meanElev', 'paleoData_variableName', 'paleoData_units',
'paleoData_proxy', 'paleoData_proxyGeneral', 'time_variableName',
'compilationName', 'TSID'],
dtype='object')
df_metadata.to_csv('metadata.csv')
Step 4: Slice for the time frame of interest#
We can use Pyleoclim to do this for us
time_slice = []
value_slice = []
for _,row in df_nodup.iterrows():
ts=pyleo.Series(time = row['time_values'],
value = row ['paleoData_values'],
time_name = row ['time_variableName'],
time_unit = row ['time_units'],
value_name = row ['paleoData_variableName'],
value_unit = row['paleoData_units'], verbose=False)
ts_slice = ts.slice([0,2000])
time_slice.append(ts_slice.time)
value_slice.append(ts_slice.value)
df_nodup['time_values_slice']=time_slice
df_nodup['paleoData_values_slice'] = value_slice
df_nodup.head()
dataSetName | archiveType | geo_meanLat | geo_meanLon | geo_meanElev | paleoData_variableName | paleoData_values | paleoData_units | paleoData_proxy | paleoData_proxyGeneral | time_variableName | time_values | time_units | compilationName | TSID | QAlabel | time_values_slice | paleoData_values_slice | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | LS16STCL | LakeSediment | 50.8300 | -116.3900 | 1126.0 | d18O | [-7.81, -5.91, -9.03, -5.35, -5.61, -5.98, -5.... | permil | d18O | NaN | year | [2009.0, 2008.3, 2007.8, 2007.4, 2007.0, 2006.... | AD | iso2k | LPD7dc5b9ba | True | [0.299999999999955, 2.90000000000009, 5.700000... | [-5.36, -5.34, -5.12, -5.19, -5.34, -5.32, -6.... |
1 | CO00URMA | Coral | 0.9330 | 173.0000 | 6.0 | d18O | [-4.8011, -4.725, -4.6994, -4.86, -5.0886, -5.... | permil | d18O | NaN | year | [1994.5, 1994.33, 1994.17, 1994.0, 1993.83, 19... | AD | iso2k | Ocean2kHR_177 | True | [1840.0, 1840.17, 1840.33, 1840.5, 1840.67, 18... | [-4.6914, -4.7834, -4.5927, -4.3615, -4.315, -... |
4 | CO05KUBE | Coral | 32.4670 | -64.7000 | -12.0 | d18O | [-4.15, -3.66, -3.69, -4.07, -3.95, -4.12, -3.... | permil | d18O | NaN | year | [1983.21, 1983.13, 1983.04, 1982.96, 1982.88, ... | AD | iso2k | Ocean2kHR_105 | True | [1825.21, 1825.29, 1825.38, 1825.46, 1825.54, ... | [-3.1, -3.2, -3.37, -3.79, -3.94, -3.88, -3.74... |
5 | IC13THQU | GlacierIce | -13.9333 | -70.8333 | 5670.0 | d18O | [-18.5905, -16.3244, -16.2324, -17.0112, -18.6... | permil | d18O | NaN | year | [2009, 2008, 2007, 2006, 2005, 2004, 2003, 200... | AD | iso2k | SAm_035 | True | [226.0, 227.0, 228.0, 229.0, 230.0, 231.0, 232... | [-18.12, -17.48, -17.33, -17.84, -18.45, -18.8... |
6 | CO01TUNG | Coral | -5.2170 | 145.8170 | -3.0 | d18O | [-4.827, -4.786, -4.693, -4.852, -4.991, -4.90... | permil | d18O | NaN | year | [1993.042, 1992.792, 1992.542, 1992.292, 1992.... | AD | iso2k | Ocean2kHR_140 | True | [1880.792, 1881.042, 1881.292, 1881.542, 1881.... | [-4.801, -4.94, -4.906, -4.792, -4.923, -5.023... |
Step 4: Gaussianize#
Let’s have Pyleoclim help us with that
value_gaussianize = []
for _,row in df_nodup.iterrows():
ts=pyleo.Series(time = row['time_values_slice'],
value = row ['paleoData_values_slice'],
time_name = row ['time_variableName'],
time_unit = row ['time_units'],
value_name = row ['paleoData_variableName'],
value_unit = row['paleoData_units'], verbose=False)
ts_g = ts.gaussianize()
value_gaussianize.append(ts_g.value)
df_nodup['paleoData_values_slice_gauss'] = value_gaussianize
df_nodup.head()
dataSetName | archiveType | geo_meanLat | geo_meanLon | geo_meanElev | paleoData_variableName | paleoData_values | paleoData_units | paleoData_proxy | paleoData_proxyGeneral | time_variableName | time_values | time_units | compilationName | TSID | QAlabel | time_values_slice | paleoData_values_slice | paleoData_values_slice_gauss | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | LS16STCL | LakeSediment | 50.8300 | -116.3900 | 1126.0 | d18O | [-7.81, -5.91, -9.03, -5.35, -5.61, -5.98, -5.... | permil | d18O | NaN | year | [2009.0, 2008.3, 2007.8, 2007.4, 2007.0, 2006.... | AD | iso2k | LPD7dc5b9ba | True | [0.299999999999955, 2.90000000000009, 5.700000... | [-5.36, -5.34, -5.12, -5.19, -5.34, -5.32, -6.... | [-0.2130875894530718, -0.17422655914690632, 0.... |
1 | CO00URMA | Coral | 0.9330 | 173.0000 | 6.0 | d18O | [-4.8011, -4.725, -4.6994, -4.86, -5.0886, -5.... | permil | d18O | NaN | year | [1994.5, 1994.33, 1994.17, 1994.0, 1993.83, 19... | AD | iso2k | Ocean2kHR_177 | True | [1840.0, 1840.17, 1840.33, 1840.5, 1840.67, 18... | [-4.6914, -4.7834, -4.5927, -4.3615, -4.315, -... | [-0.9811705980359124, -1.2651460843379647, -0.... |
4 | CO05KUBE | Coral | 32.4670 | -64.7000 | -12.0 | d18O | [-4.15, -3.66, -3.69, -4.07, -3.95, -4.12, -3.... | permil | d18O | NaN | year | [1983.21, 1983.13, 1983.04, 1982.96, 1982.88, ... | AD | iso2k | Ocean2kHR_105 | True | [1825.21, 1825.29, 1825.38, 1825.46, 1825.54, ... | [-3.1, -3.2, -3.37, -3.79, -3.94, -3.88, -3.74... | [1.328615981030611, 0.9458448462285661, 0.4131... |
5 | IC13THQU | GlacierIce | -13.9333 | -70.8333 | 5670.0 | d18O | [-18.5905, -16.3244, -16.2324, -17.0112, -18.6... | permil | d18O | NaN | year | [2009, 2008, 2007, 2006, 2005, 2004, 2003, 200... | AD | iso2k | SAm_035 | True | [226.0, 227.0, 228.0, 229.0, 230.0, 231.0, 232... | [-18.12, -17.48, -17.33, -17.84, -18.45, -18.8... | [-0.24533483270695292, 0.3501885714345531, 0.5... |
6 | CO01TUNG | Coral | -5.2170 | 145.8170 | -3.0 | d18O | [-4.827, -4.786, -4.693, -4.852, -4.991, -4.90... | permil | d18O | NaN | year | [1993.042, 1992.792, 1992.542, 1992.292, 1992.... | AD | iso2k | Ocean2kHR_140 | True | [1880.792, 1881.042, 1881.292, 1881.542, 1881.... | [-4.801, -4.94, -4.906, -4.792, -4.923, -5.023... | [0.7315480859971977, 0.03064136195287292, 0.21... |
Step 5: Create the composite#
Step 5a: Clean up the proxy/variable names#
temp = df_nodup[['archiveType','paleoData_variableName']]
temp.groupby(['archiveType','paleoData_variableName'], as_index=False).value_counts()
archiveType | paleoData_variableName | count | |
---|---|---|---|
0 | Coral | d18O | 45 |
1 | GlacierIce | d18O | 1 |
2 | LakeSediment | d18O | 3 |
3 | LakeSediment | d2H | 1 |
4 | Sclerosponge | d18O | 3 |
5 | bivalve | d18O | 1 |
6 | borehole | temperature | 2 |
7 | coral | Sr_Ca | 24 |
8 | coral | calcification | 2 |
9 | coral | composite | 6 |
10 | coral | d18O | 23 |
11 | coral | temperature | 1 |
12 | documents | temperature | 14 |
13 | glacier ice | d18O | 6 |
14 | glacier ice | dD | 1 |
15 | hybrid | temperature | 1 |
16 | lake sediment | RABD660_670 | 1 |
17 | lake sediment | X_radiograph_dark_layer | 1 |
18 | lake sediment | temperature | 26 |
19 | lake sediment | thickness | 3 |
20 | marine sediment | d18O | 1 |
21 | marine sediment | temperature | 52 |
22 | sclerosponge | Sr_Ca | 4 |
23 | sclerosponge | d18O | 3 |
24 | speleothem | d18O | 4 |
25 | tree | MXD | 52 |
26 | tree | density | 4 |
27 | tree | temperature | 6 |
28 | tree | trsgi | 321 |
Get the standard archive and variable names:
archive_std = pd.read_csv('archiveType.csv')
df_archive = archive_std[['lipdName','synonym']].reset_index()
syn = []
for _,row in df_archive.iterrows():
syn.append(row['synonym'].lower().replace(' ',""))
df_archive['synonym_reg']=syn
var_std=pd.read_csv('paleoData_variableName.csv')
df_var = var_std[['lipdName','synonym']].reset_index()
syn_var=[]
for _,row in df_var.iterrows():
try:
syn_var.append(row['synonym'].lower().replace(' ',"").replace('/',"").replace('_',""))
except:
syn_var.append(row['synonym'])
df_var['synonym_reg']=syn_var
#let's do the archive first
archiveType_lipd = []
for _, row in df_nodup.iterrows():
match = row['archiveType'].lower().replace(' ',"").replace('/',"").replace('_',"")
f = df_archive[df_archive['synonym_reg']==match]
if type(f) == pd.core.frame.DataFrame and not f.empty:
archiveType_lipd.append(f['lipdName'].iloc[0])
elif type(f) == pd.core.series.Series:
archiveType_lipd.append(f['lipdName'])
else:
archiveType_lipd.append(row['archiveType'])
#let's work on varname
variableName = []
for _, row in df_nodup.iterrows():
match = row['paleoData_variableName'].lower().replace(' ',"").replace('/',"").replace('_',"")
f = df_var[df_var['synonym_reg']==match]
if type(f) == pd.core.frame.DataFrame and not f.empty:
variableName.append(f['lipdName'].iloc[0])
elif type(f) == pd.core.series.Series:
variableName.append(f['lipdName'])
else:
variableName.append(row['archiveType'])
ptype = []
for idx,item in enumerate(variableName):
ptype.append(archiveType_lipd[idx]+'.'+item)
Put it in our DataFrame:
df_nodup['ptype']=ptype
Step 5b: Set the style for the figures#
colors_dict = {}
for idx, item in enumerate(set(ptype)):
if 'marinesediment' in item.lower():
colors_dict[item] = sns.xkcd_rgb['brown']
elif 'lakesediment' in item.lower():
colors_dict[item]= sns.xkcd_rgb['maroon']
elif 'coral' in item.lower():
colors_dict[item]= sns.xkcd_rgb['orange']
elif 'ice' in item.lower():
colors_dict[item]= sns.xkcd_rgb['sky blue']
elif 'borehole' in item.lower():
colors_dict[item]= sns.xkcd_rgb['dark blue']
elif 'sclerosponge' in item.lower():
colors_dict[item]= sns.xkcd_rgb['amber']
elif 'speleothem' in item.lower():
colors_dict[item]= sns.xkcd_rgb['mauve']
elif 'wood' in item.lower():
colors_dict[item]= sns.xkcd_rgb['forest green']
elif 'bivalve' in item.lower():
colors_dict[item]= sns.xkcd_rgb['peach']
else:
colors_dict[item]= 'k'
markers_dict = {}
for idx, item in enumerate(set(ptype)):
if 'temperature' in item.lower():
markers_dict[item] = 'P'
elif 'srca' in item.lower().replace('/',''):
markers_dict[item]= 'X'
elif 'd18o' in item.lower():
markers_dict[item] = 'o'
elif 'calcification' in item.lower():
markers_dict[item] = 'H'
elif 'composite' in item.lower():
markers_dict[item] = '<'
elif 'd2h' in item.lower():
markers_dict[item] = 'd'
elif 'reflectance' in item.lower():
markers_dict[item] = '>'
elif 'thickness' in item.lower():
markers_dict[item] = 'h'
elif 'mxd' in item.lower():
markers_dict[item] = '8'
elif 'density' in item.lower():
markers_dict[item] = '^'
elif 'width' in item.lower():
markers_dict[item] = 'v'
else:
markers_dict[item] = 's'
Reset the plotting style:
cfr.visual.STYLE.colors_dict = colors_dict
cfr.visual.STYLE.markers_dict = markers_dict
Step 5c: Create the Proxy DataBase#
Let’s try to get one ProxyRecord into cfr
:
row = df_nodup.iloc[0,:]
f = cfr.ProxyRecord(pid = row['TSID'],
time = row['time_values_slice'],
value = row['paleoData_values_slice_gauss'],
lat = row['geo_meanLat'],
lon = row['geo_meanLon'],
elev = row['geo_meanElev'],
ptype = row['ptype'],
tags = None,
value_name = row['paleoData_variableName'],
value_unit = row['paleoData_units'],
time_name = row['time_units'],
seasonality = None)
f.plot()
(<Figure size 1200x400 with 2 Axes>,
{'ts': <AxesSubplot: title={'center': 'LPD7dc5b9ba (LakeSediment.d18O) @ (lat:50.83, lon:243.61)'}, xlabel='AD [yr]', ylabel='d18O [permil]'>,
'map': <GeoAxesSubplot: >})
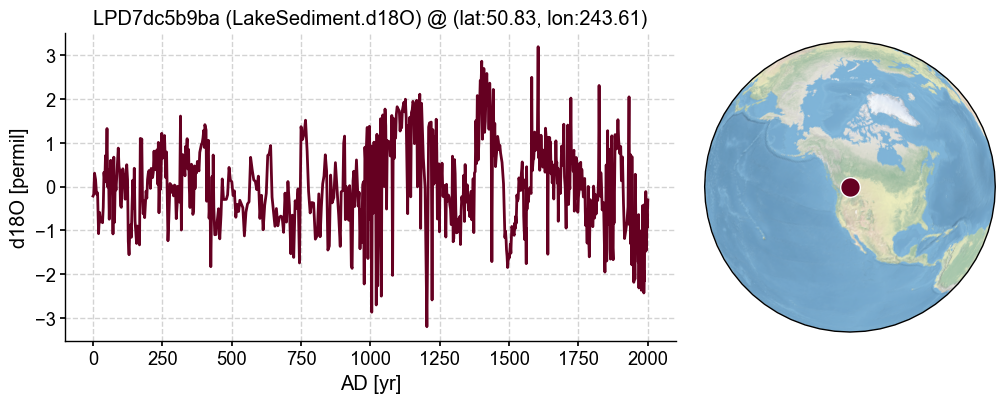
Now let’s try to get the proxy database sorted out:
proxy_dict = {}
for _,row in df_nodup.iterrows():
proxy_dict[row['TSID']] = cfr.ProxyRecord(pid = row['TSID'],
time = row['time_values_slice'],
value = row['paleoData_values_slice_gauss'],
lat = row['geo_meanLat'],
lon = row['geo_meanLon'],
elev = row['geo_meanElev'],
ptype = row['ptype'],
tags = None,
value_name = row['paleoData_variableName'],
value_unit = row['paleoData_units'],
time_name = row['time_units'],
seasonality = None)
pdb = cfr.ProxyDatabase(proxy_dict)
fig, ax = pdb.plot()
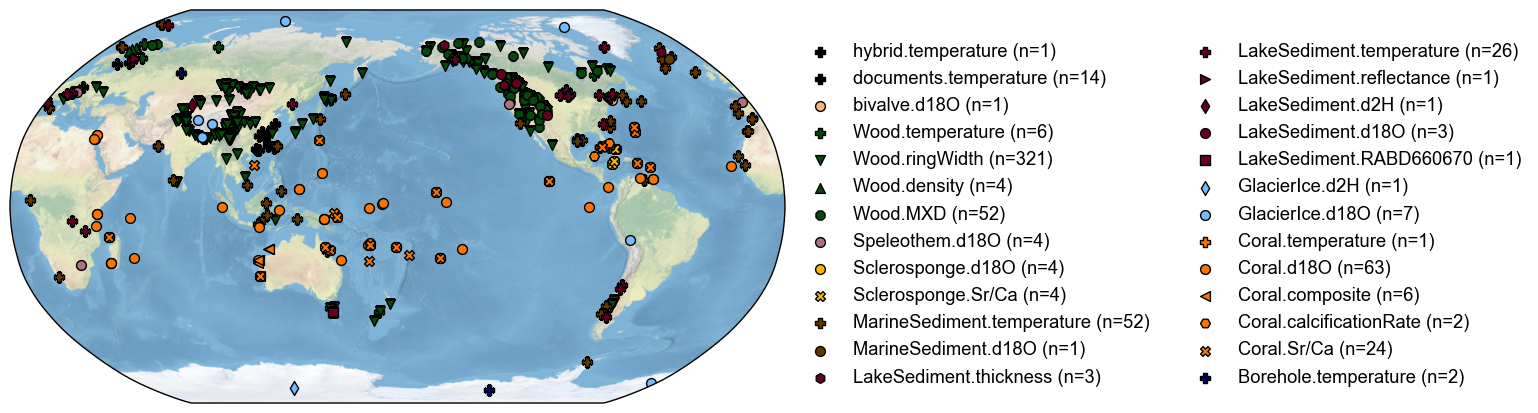
Step 5d: Calibrate against instrumental data#
import xarray as xr
ds = xr.open_dataset('./data/HadCRUT.5.0.1.0.analysis.anomalies.ensemble_mean.nc')
ds
<xarray.Dataset> Dimensions: (time: 2071, latitude: 36, longitude: 72, bnds: 2) Coordinates: * time (time) datetime64[ns] 1850-01-16T12:00:00 ... 2022-07-1... * latitude (latitude) float64 -87.5 -82.5 -77.5 ... 77.5 82.5 87.5 * longitude (longitude) float64 -177.5 -172.5 -167.5 ... 172.5 177.5 realization int64 ... Dimensions without coordinates: bnds Data variables: tas_mean (time, latitude, longitude) float64 ... time_bnds (time, bnds) datetime64[ns] ... latitude_bnds (latitude, bnds) float64 ... longitude_bnds (longitude, bnds) float64 ... realization_bnds (bnds) int64 ... Attributes: comment: 2m air temperature over land blended with sea water tempera... history: Data set built at: 2022-09-06T13:35:49+00:00 institution: Met Office Hadley Centre / Climatic Research Unit, Universi... licence: HadCRUT5 is licensed under the Open Government Licence v3.0... reference: C. P. Morice, J. J. Kennedy, N. A. Rayner, J. P. Winn, E. H... source: CRUTEM.5.0.1.0 HadSST.4.0.0.0 title: HadCRUT.5.0.1.0 blended land air temperature and sea-surfac... version: HadCRUT.5.0.1.0 Conventions: CF-1.7
obs = cfr.ClimateField().load_nc(
'./data/HadCRUT.5.0.1.0.analysis.anomalies.ensemble_mean.nc',
vn='tas_mean', lat_name='latitude', lon_name='longitude',
)
obs = obs.rename('tas')
Step 5e: make the composite#
pdb.make_composite(obs,bin_width=10)
Analyzing ProxyRecord: 0%| | 0/605 [00:00<?, ?it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 0%| | 1/605 [00:00<02:57, 3.40it/s]
Analyzing ProxyRecord: 0%| | 2/605 [00:00<02:36, 3.86it/s]
Analyzing ProxyRecord: 0%|▏ | 3/605 [00:00<02:26, 4.11it/s]
Analyzing ProxyRecord: 1%|▏ | 4/605 [00:00<02:24, 4.16it/s]
Analyzing ProxyRecord: 1%|▏ | 5/605 [00:01<02:18, 4.34it/s]
Analyzing ProxyRecord: 1%|▎ | 6/605 [00:01<02:16, 4.38it/s]
Analyzing ProxyRecord: 1%|▎ | 7/605 [00:01<02:17, 4.35it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 1%|▎ | 8/605 [00:01<02:15, 4.41it/s]
Analyzing ProxyRecord: 1%|▍ | 9/605 [00:02<02:14, 4.42it/s]
Analyzing ProxyRecord: 2%|▍ | 10/605 [00:02<02:14, 4.43it/s]
Analyzing ProxyRecord: 2%|▍ | 11/605 [00:02<02:16, 4.34it/s]
Analyzing ProxyRecord: 2%|▌ | 12/605 [00:02<02:18, 4.28it/s]
Analyzing ProxyRecord: 2%|▌ | 13/605 [00:03<02:17, 4.29it/s]
Analyzing ProxyRecord: 2%|▌ | 14/605 [00:03<02:16, 4.34it/s]
Analyzing ProxyRecord: 2%|▋ | 15/605 [00:03<02:14, 4.39it/s]
Analyzing ProxyRecord: 3%|▋ | 16/605 [00:03<02:11, 4.49it/s]
Analyzing ProxyRecord: 3%|▊ | 17/605 [00:03<02:11, 4.48it/s]
Analyzing ProxyRecord: 3%|▊ | 18/605 [00:04<02:10, 4.51it/s]
Analyzing ProxyRecord: 3%|▊ | 19/605 [00:04<02:08, 4.55it/s]
Analyzing ProxyRecord: 3%|▉ | 20/605 [00:04<02:08, 4.55it/s]
Analyzing ProxyRecord: 3%|▉ | 21/605 [00:04<02:09, 4.50it/s]
Analyzing ProxyRecord: 4%|▉ | 22/605 [00:05<02:09, 4.51it/s]
Analyzing ProxyRecord: 4%|█ | 23/605 [00:05<02:08, 4.52it/s]
Analyzing ProxyRecord: 4%|█ | 24/605 [00:05<02:08, 4.53it/s]
Analyzing ProxyRecord: 4%|█ | 25/605 [00:05<02:05, 4.61it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 4%|█▏ | 26/605 [00:05<02:04, 4.64it/s]
Analyzing ProxyRecord: 4%|█▏ | 27/605 [00:06<02:02, 4.70it/s]
Analyzing ProxyRecord: 5%|█▏ | 28/605 [00:06<02:03, 4.68it/s]
Analyzing ProxyRecord: 5%|█▎ | 29/605 [00:06<02:04, 4.62it/s]
Analyzing ProxyRecord: 5%|█▎ | 30/605 [00:06<02:04, 4.62it/s]
Analyzing ProxyRecord: 5%|█▍ | 31/605 [00:06<02:05, 4.57it/s]
Analyzing ProxyRecord: 5%|█▍ | 32/605 [00:07<02:07, 4.50it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 5%|█▍ | 33/605 [00:07<02:06, 4.52it/s]
Analyzing ProxyRecord: 6%|█▌ | 34/605 [00:07<02:04, 4.58it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 6%|█▌ | 35/605 [00:07<02:03, 4.63it/s]
Analyzing ProxyRecord: 6%|█▌ | 36/605 [00:08<02:03, 4.61it/s]
Analyzing ProxyRecord: 6%|█▋ | 37/605 [00:08<02:04, 4.56it/s]
Analyzing ProxyRecord: 6%|█▋ | 38/605 [00:08<02:05, 4.52it/s]
Analyzing ProxyRecord: 6%|█▋ | 39/605 [00:08<02:03, 4.57it/s]
Analyzing ProxyRecord: 7%|█▊ | 40/605 [00:08<02:03, 4.57it/s]
Analyzing ProxyRecord: 7%|█▊ | 41/605 [00:09<02:01, 4.64it/s]
Analyzing ProxyRecord: 7%|█▊ | 42/605 [00:09<02:02, 4.58it/s]
Analyzing ProxyRecord: 7%|█▉ | 43/605 [00:09<02:02, 4.59it/s]
Analyzing ProxyRecord: 7%|█▉ | 44/605 [00:09<02:03, 4.53it/s]
Analyzing ProxyRecord: 7%|██ | 45/605 [00:10<02:02, 4.56it/s]
Analyzing ProxyRecord: 8%|██ | 46/605 [00:10<02:02, 4.56it/s]
Analyzing ProxyRecord: 8%|██ | 47/605 [00:10<02:02, 4.54it/s]
Analyzing ProxyRecord: 8%|██▏ | 48/605 [00:10<02:00, 4.60it/s]
Analyzing ProxyRecord: 8%|██▏ | 49/605 [00:10<01:59, 4.66it/s]
Analyzing ProxyRecord: 8%|██▏ | 50/605 [00:11<02:05, 4.42it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 8%|██▎ | 51/605 [00:11<02:09, 4.27it/s]
Analyzing ProxyRecord: 9%|██▎ | 52/605 [00:11<02:09, 4.26it/s]
Analyzing ProxyRecord: 9%|██▎ | 53/605 [00:11<02:07, 4.34it/s]
Analyzing ProxyRecord: 9%|██▍ | 54/605 [00:12<02:04, 4.42it/s]
Analyzing ProxyRecord: 9%|██▍ | 55/605 [00:12<02:01, 4.53it/s]
Analyzing ProxyRecord: 9%|██▍ | 56/605 [00:12<01:59, 4.60it/s]
Analyzing ProxyRecord: 9%|██▌ | 57/605 [00:12<01:57, 4.66it/s]
Analyzing ProxyRecord: 10%|██▌ | 58/605 [00:12<01:56, 4.69it/s]
Analyzing ProxyRecord: 10%|██▋ | 59/605 [00:13<01:55, 4.71it/s]
Analyzing ProxyRecord: 10%|██▋ | 60/605 [00:13<01:54, 4.75it/s]
Analyzing ProxyRecord: 10%|██▋ | 61/605 [00:13<01:54, 4.76it/s]
Analyzing ProxyRecord: 10%|██▊ | 62/605 [00:13<01:52, 4.81it/s]
Analyzing ProxyRecord: 10%|██▊ | 63/605 [00:13<01:51, 4.85it/s]
Analyzing ProxyRecord: 11%|██▊ | 64/605 [00:14<01:52, 4.80it/s]
Analyzing ProxyRecord: 11%|██▉ | 65/605 [00:14<01:52, 4.81it/s]
Analyzing ProxyRecord: 11%|██▉ | 66/605 [00:14<01:52, 4.81it/s]
Analyzing ProxyRecord: 11%|██▉ | 67/605 [00:14<01:51, 4.82it/s]
Analyzing ProxyRecord: 11%|███ | 68/605 [00:14<01:51, 4.82it/s]
Analyzing ProxyRecord: 11%|███ | 69/605 [00:15<01:50, 4.84it/s]
Analyzing ProxyRecord: 12%|███ | 70/605 [00:15<01:49, 4.87it/s]
Analyzing ProxyRecord: 12%|███▏ | 71/605 [00:15<01:50, 4.83it/s]
Analyzing ProxyRecord: 12%|███▏ | 72/605 [00:15<01:50, 4.82it/s]
Analyzing ProxyRecord: 12%|███▎ | 73/605 [00:16<01:50, 4.83it/s]
Analyzing ProxyRecord: 12%|███▎ | 74/605 [00:16<01:50, 4.81it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 12%|███▎ | 75/605 [00:16<01:51, 4.75it/s]
Analyzing ProxyRecord: 13%|███▍ | 76/605 [00:16<01:50, 4.77it/s]
Analyzing ProxyRecord: 13%|███▍ | 77/605 [00:16<01:50, 4.76it/s]
Analyzing ProxyRecord: 13%|███▍ | 78/605 [00:17<01:51, 4.71it/s]
Analyzing ProxyRecord: 13%|███▌ | 79/605 [00:17<01:51, 4.73it/s]
Analyzing ProxyRecord: 13%|███▌ | 80/605 [00:17<01:50, 4.74it/s]
Analyzing ProxyRecord: 13%|███▌ | 81/605 [00:17<01:50, 4.76it/s]
Analyzing ProxyRecord: 14%|███▋ | 82/605 [00:17<01:49, 4.77it/s]
Analyzing ProxyRecord: 14%|███▋ | 83/605 [00:18<01:49, 4.75it/s]
Analyzing ProxyRecord: 14%|███▋ | 84/605 [00:18<01:49, 4.76it/s]
Analyzing ProxyRecord: 14%|███▊ | 85/605 [00:18<01:50, 4.71it/s]
Analyzing ProxyRecord: 14%|███▊ | 86/605 [00:18<01:50, 4.71it/s]
Analyzing ProxyRecord: 14%|███▉ | 87/605 [00:18<01:50, 4.69it/s]
Analyzing ProxyRecord: 15%|███▉ | 88/605 [00:19<01:48, 4.75it/s]
Analyzing ProxyRecord: 15%|███▉ | 89/605 [00:19<01:48, 4.78it/s]
Analyzing ProxyRecord: 15%|████ | 90/605 [00:19<01:47, 4.80it/s]
Analyzing ProxyRecord: 15%|████ | 91/605 [00:19<01:47, 4.78it/s]
Analyzing ProxyRecord: 15%|████ | 92/605 [00:20<01:46, 4.83it/s]
Analyzing ProxyRecord: 15%|████▏ | 93/605 [00:20<01:46, 4.81it/s]
Analyzing ProxyRecord: 16%|████▏ | 94/605 [00:20<01:46, 4.80it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 16%|████▏ | 95/605 [00:20<01:46, 4.77it/s]
Analyzing ProxyRecord: 16%|████▎ | 96/605 [00:20<01:46, 4.77it/s]
Analyzing ProxyRecord: 16%|████▎ | 97/605 [00:21<01:46, 4.79it/s]
Analyzing ProxyRecord: 16%|████▎ | 98/605 [00:21<01:46, 4.78it/s]
Analyzing ProxyRecord: 16%|████▍ | 99/605 [00:21<01:45, 4.78it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 17%|████▎ | 100/605 [00:21<01:46, 4.76it/s]
Analyzing ProxyRecord: 17%|████▎ | 101/605 [00:21<01:45, 4.76it/s]
Analyzing ProxyRecord: 17%|████▍ | 102/605 [00:22<01:45, 4.75it/s]
Analyzing ProxyRecord: 17%|████▍ | 103/605 [00:22<01:45, 4.76it/s]
Analyzing ProxyRecord: 17%|████▍ | 104/605 [00:22<01:45, 4.76it/s]
Analyzing ProxyRecord: 17%|████▌ | 105/605 [00:22<01:45, 4.75it/s]
Analyzing ProxyRecord: 18%|████▌ | 106/605 [00:22<01:45, 4.75it/s]
Analyzing ProxyRecord: 18%|████▌ | 107/605 [00:23<01:44, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 18%|████▋ | 108/605 [00:23<01:45, 4.72it/s]
Analyzing ProxyRecord: 18%|████▋ | 109/605 [00:23<01:45, 4.72it/s]
Analyzing ProxyRecord: 18%|████▋ | 110/605 [00:23<01:43, 4.77it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 18%|████▊ | 111/605 [00:24<01:44, 4.75it/s]
Analyzing ProxyRecord: 19%|████▊ | 112/605 [00:24<01:43, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 19%|████▊ | 113/605 [00:24<01:43, 4.76it/s]
Analyzing ProxyRecord: 19%|████▉ | 114/605 [00:24<01:42, 4.77it/s]
Analyzing ProxyRecord: 19%|████▉ | 115/605 [00:24<01:42, 4.77it/s]
Analyzing ProxyRecord: 19%|████▉ | 116/605 [00:25<01:41, 4.79it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 19%|█████ | 117/605 [00:25<01:42, 4.76it/s]
Analyzing ProxyRecord: 20%|█████ | 118/605 [00:25<01:41, 4.79it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 20%|█████ | 119/605 [00:25<01:41, 4.77it/s]
Analyzing ProxyRecord: 20%|█████▏ | 120/605 [00:25<01:41, 4.78it/s]
Analyzing ProxyRecord: 20%|█████▏ | 121/605 [00:26<01:40, 4.82it/s]
Analyzing ProxyRecord: 20%|█████▏ | 122/605 [00:26<01:40, 4.79it/s]
Analyzing ProxyRecord: 20%|█████▎ | 123/605 [00:26<01:40, 4.80it/s]
Analyzing ProxyRecord: 20%|█████▎ | 124/605 [00:26<01:39, 4.81it/s]
Analyzing ProxyRecord: 21%|█████▎ | 125/605 [00:26<01:39, 4.84it/s]
Analyzing ProxyRecord: 21%|█████▍ | 126/605 [00:27<01:39, 4.82it/s]
Analyzing ProxyRecord: 21%|█████▍ | 127/605 [00:27<01:39, 4.80it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 21%|█████▌ | 128/605 [00:27<01:39, 4.78it/s]
Analyzing ProxyRecord: 21%|█████▌ | 129/605 [00:27<01:40, 4.75it/s]
Analyzing ProxyRecord: 21%|█████▌ | 130/605 [00:27<01:40, 4.73it/s]
Analyzing ProxyRecord: 22%|█████▋ | 131/605 [00:28<01:39, 4.75it/s]
Analyzing ProxyRecord: 22%|█████▋ | 132/605 [00:28<01:40, 4.69it/s]
Analyzing ProxyRecord: 22%|█████▋ | 133/605 [00:28<01:40, 4.68it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 22%|█████▊ | 134/605 [00:28<01:40, 4.68it/s]
Analyzing ProxyRecord: 22%|█████▊ | 135/605 [00:29<01:39, 4.71it/s]
Analyzing ProxyRecord: 22%|█████▊ | 136/605 [00:29<01:38, 4.76it/s]
Analyzing ProxyRecord: 23%|█████▉ | 137/605 [00:29<01:37, 4.78it/s]
Analyzing ProxyRecord: 23%|█████▉ | 138/605 [00:29<01:37, 4.78it/s]
Analyzing ProxyRecord: 23%|█████▉ | 139/605 [00:29<01:37, 4.79it/s]
Analyzing ProxyRecord: 23%|██████ | 140/605 [00:30<01:37, 4.78it/s]
Analyzing ProxyRecord: 23%|██████ | 141/605 [00:30<01:36, 4.79it/s]
Analyzing ProxyRecord: 23%|██████ | 142/605 [00:30<01:36, 4.78it/s]
Analyzing ProxyRecord: 24%|██████▏ | 143/605 [00:30<01:36, 4.80it/s]
Analyzing ProxyRecord: 24%|██████▏ | 144/605 [00:30<01:35, 4.83it/s]
Analyzing ProxyRecord: 24%|██████▏ | 145/605 [00:31<01:35, 4.81it/s]
Analyzing ProxyRecord: 24%|██████▎ | 146/605 [00:31<01:35, 4.79it/s]
Analyzing ProxyRecord: 24%|██████▎ | 147/605 [00:31<01:35, 4.78it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 24%|██████▎ | 148/605 [00:31<01:35, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 25%|██████▍ | 149/605 [00:31<01:36, 4.74it/s]
Analyzing ProxyRecord: 25%|██████▍ | 150/605 [00:32<01:35, 4.75it/s]
Analyzing ProxyRecord: 25%|██████▍ | 151/605 [00:32<01:34, 4.78it/s]
Analyzing ProxyRecord: 25%|██████▌ | 152/605 [00:32<01:34, 4.80it/s]
Analyzing ProxyRecord: 25%|██████▌ | 153/605 [00:32<01:33, 4.82it/s]
Analyzing ProxyRecord: 25%|██████▌ | 154/605 [00:33<01:33, 4.80it/s]
Analyzing ProxyRecord: 26%|██████▋ | 155/605 [00:33<01:33, 4.83it/s]
Analyzing ProxyRecord: 26%|██████▋ | 156/605 [00:33<01:33, 4.82it/s]
Analyzing ProxyRecord: 26%|██████▋ | 157/605 [00:33<01:32, 4.85it/s]
Analyzing ProxyRecord: 26%|██████▊ | 158/605 [00:33<01:32, 4.85it/s]
Analyzing ProxyRecord: 26%|██████▊ | 159/605 [00:34<01:32, 4.84it/s]
Analyzing ProxyRecord: 26%|██████▉ | 160/605 [00:34<01:31, 4.85it/s]
Analyzing ProxyRecord: 27%|██████▉ | 161/605 [00:34<01:32, 4.82it/s]
Analyzing ProxyRecord: 27%|██████▉ | 162/605 [00:34<01:32, 4.81it/s]
Analyzing ProxyRecord: 27%|███████ | 163/605 [00:34<01:32, 4.78it/s]
Analyzing ProxyRecord: 27%|███████ | 164/605 [00:35<01:32, 4.76it/s]
Analyzing ProxyRecord: 27%|███████ | 165/605 [00:35<01:32, 4.77it/s]
Analyzing ProxyRecord: 27%|███████▏ | 166/605 [00:35<01:31, 4.78it/s]
Analyzing ProxyRecord: 28%|███████▏ | 167/605 [00:35<01:31, 4.79it/s]
Analyzing ProxyRecord: 28%|███████▏ | 168/605 [00:35<01:31, 4.78it/s]
Analyzing ProxyRecord: 28%|███████▎ | 169/605 [00:36<01:31, 4.79it/s]
Analyzing ProxyRecord: 28%|███████▎ | 170/605 [00:36<01:30, 4.78it/s]
Analyzing ProxyRecord: 28%|███████▎ | 171/605 [00:36<01:31, 4.77it/s]
Analyzing ProxyRecord: 28%|███████▍ | 172/605 [00:36<01:30, 4.79it/s]
Analyzing ProxyRecord: 29%|███████▍ | 173/605 [00:36<01:29, 4.82it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 29%|███████▍ | 174/605 [00:37<01:30, 4.77it/s]
Analyzing ProxyRecord: 29%|███████▌ | 175/605 [00:37<01:30, 4.76it/s]
Analyzing ProxyRecord: 29%|███████▌ | 176/605 [00:37<01:29, 4.80it/s]
Analyzing ProxyRecord: 29%|███████▌ | 177/605 [00:37<01:29, 4.78it/s]
Analyzing ProxyRecord: 29%|███████▋ | 178/605 [00:38<01:29, 4.78it/s]
Analyzing ProxyRecord: 30%|███████▋ | 179/605 [00:38<01:29, 4.77it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 30%|███████▋ | 180/605 [00:38<01:28, 4.80it/s]
Analyzing ProxyRecord: 30%|███████▊ | 181/605 [00:38<01:28, 4.80it/s]
Analyzing ProxyRecord: 30%|███████▊ | 182/605 [00:38<01:28, 4.80it/s]
Analyzing ProxyRecord: 30%|███████▊ | 183/605 [00:39<01:27, 4.80it/s]
Analyzing ProxyRecord: 30%|███████▉ | 184/605 [00:39<01:27, 4.80it/s]
Analyzing ProxyRecord: 31%|███████▉ | 185/605 [00:39<01:26, 4.83it/s]
Analyzing ProxyRecord: 31%|███████▉ | 186/605 [00:39<01:27, 4.81it/s]
Analyzing ProxyRecord: 31%|████████ | 187/605 [00:39<01:26, 4.81it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 31%|████████ | 188/605 [00:40<01:26, 4.79it/s]
Analyzing ProxyRecord: 31%|████████ | 189/605 [00:40<01:27, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 31%|████████▏ | 190/605 [00:40<01:27, 4.75it/s]
Analyzing ProxyRecord: 32%|████████▏ | 191/605 [00:40<01:26, 4.77it/s]
Analyzing ProxyRecord: 32%|████████▎ | 192/605 [00:40<01:26, 4.76it/s]
Analyzing ProxyRecord: 32%|████████▎ | 193/605 [00:41<01:26, 4.79it/s]
Analyzing ProxyRecord: 32%|████████▎ | 194/605 [00:41<01:25, 4.81it/s]
Analyzing ProxyRecord: 32%|████████▍ | 195/605 [00:41<01:25, 4.81it/s]
Analyzing ProxyRecord: 32%|████████▍ | 196/605 [00:41<01:25, 4.79it/s]
Analyzing ProxyRecord: 33%|████████▍ | 197/605 [00:41<01:25, 4.76it/s]
Analyzing ProxyRecord: 33%|████████▌ | 198/605 [00:42<01:25, 4.76it/s]
Analyzing ProxyRecord: 33%|████████▌ | 199/605 [00:42<01:24, 4.80it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 33%|████████▌ | 200/605 [00:42<01:25, 4.75it/s]
Analyzing ProxyRecord: 33%|████████▋ | 201/605 [00:42<01:24, 4.77it/s]
Analyzing ProxyRecord: 33%|████████▋ | 202/605 [00:43<01:24, 4.76it/s]
Analyzing ProxyRecord: 34%|████████▋ | 203/605 [00:43<01:24, 4.74it/s]
Analyzing ProxyRecord: 34%|████████▊ | 204/605 [00:43<01:24, 4.74it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 34%|████████▊ | 205/605 [00:43<01:25, 4.70it/s]
Analyzing ProxyRecord: 34%|████████▊ | 206/605 [00:43<01:25, 4.68it/s]
Analyzing ProxyRecord: 34%|████████▉ | 207/605 [00:44<01:24, 4.69it/s]
Analyzing ProxyRecord: 34%|████████▉ | 208/605 [00:44<01:24, 4.70it/s]
Analyzing ProxyRecord: 35%|████████▉ | 209/605 [00:44<01:23, 4.72it/s]
Analyzing ProxyRecord: 35%|█████████ | 210/605 [00:44<01:23, 4.72it/s]
Analyzing ProxyRecord: 35%|█████████ | 211/605 [00:44<01:22, 4.75it/s]
Analyzing ProxyRecord: 35%|█████████ | 212/605 [00:45<01:22, 4.78it/s]
Analyzing ProxyRecord: 35%|█████████▏ | 213/605 [00:45<01:21, 4.80it/s]
Analyzing ProxyRecord: 35%|█████████▏ | 214/605 [00:45<01:21, 4.78it/s]
Analyzing ProxyRecord: 36%|█████████▏ | 215/605 [00:45<01:21, 4.77it/s]
Analyzing ProxyRecord: 36%|█████████▎ | 216/605 [00:45<01:21, 4.78it/s]
Analyzing ProxyRecord: 36%|█████████▎ | 217/605 [00:46<01:21, 4.78it/s]
Analyzing ProxyRecord: 36%|█████████▎ | 218/605 [00:46<01:21, 4.77it/s]
Analyzing ProxyRecord: 36%|█████████▍ | 219/605 [00:46<01:20, 4.77it/s]
Analyzing ProxyRecord: 36%|█████████▍ | 220/605 [00:46<01:20, 4.79it/s]
Analyzing ProxyRecord: 37%|█████████▍ | 221/605 [00:47<01:21, 4.69it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 37%|█████████▌ | 222/605 [00:47<01:21, 4.71it/s]
Analyzing ProxyRecord: 37%|█████████▌ | 223/605 [00:47<01:20, 4.72it/s]
Analyzing ProxyRecord: 37%|█████████▋ | 224/605 [00:47<01:20, 4.73it/s]
Analyzing ProxyRecord: 37%|█████████▋ | 225/605 [00:47<01:20, 4.74it/s]
Analyzing ProxyRecord: 37%|█████████▋ | 226/605 [00:48<01:19, 4.75it/s]
Analyzing ProxyRecord: 38%|█████████▊ | 227/605 [00:48<01:19, 4.77it/s]
Analyzing ProxyRecord: 38%|█████████▊ | 228/605 [00:48<01:18, 4.77it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 38%|█████████▊ | 229/605 [00:48<01:18, 4.77it/s]
Analyzing ProxyRecord: 38%|█████████▉ | 230/605 [00:48<01:19, 4.74it/s]
Analyzing ProxyRecord: 38%|█████████▉ | 231/605 [00:49<01:18, 4.76it/s]
Analyzing ProxyRecord: 38%|█████████▉ | 232/605 [00:49<01:18, 4.78it/s]
Analyzing ProxyRecord: 39%|██████████ | 233/605 [00:49<01:17, 4.79it/s]
Analyzing ProxyRecord: 39%|██████████ | 234/605 [00:49<01:17, 4.77it/s]
Analyzing ProxyRecord: 39%|██████████ | 235/605 [00:49<01:17, 4.80it/s]
Analyzing ProxyRecord: 39%|██████████▏ | 236/605 [00:50<01:17, 4.79it/s]
Analyzing ProxyRecord: 39%|██████████▏ | 237/605 [00:50<01:30, 4.09it/s]
Analyzing ProxyRecord: 39%|██████████▏ | 238/605 [00:50<01:26, 4.26it/s]
Analyzing ProxyRecord: 40%|██████████▎ | 239/605 [00:50<01:22, 4.43it/s]
Analyzing ProxyRecord: 40%|██████████▎ | 240/605 [00:51<01:20, 4.54it/s]
Analyzing ProxyRecord: 40%|██████████▎ | 241/605 [00:51<01:19, 4.60it/s]
Analyzing ProxyRecord: 40%|██████████▍ | 242/605 [00:51<01:17, 4.66it/s]
Analyzing ProxyRecord: 40%|██████████▍ | 243/605 [00:51<01:17, 4.69it/s]
Analyzing ProxyRecord: 40%|██████████▍ | 244/605 [00:51<01:16, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 40%|██████████▌ | 245/605 [00:52<01:16, 4.72it/s]
Analyzing ProxyRecord: 41%|██████████▌ | 246/605 [00:52<01:15, 4.74it/s]
Analyzing ProxyRecord: 41%|██████████▌ | 247/605 [00:52<01:15, 4.74it/s]
Analyzing ProxyRecord: 41%|██████████▋ | 248/605 [00:52<01:15, 4.76it/s]
Analyzing ProxyRecord: 41%|██████████▋ | 249/605 [00:53<01:14, 4.77it/s]
Analyzing ProxyRecord: 41%|██████████▋ | 250/605 [00:53<01:13, 4.80it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 41%|██████████▊ | 251/605 [00:53<01:14, 4.76it/s]
Analyzing ProxyRecord: 42%|██████████▊ | 252/605 [00:53<01:14, 4.75it/s]
Analyzing ProxyRecord: 42%|██████████▊ | 253/605 [00:53<01:13, 4.76it/s]
Analyzing ProxyRecord: 42%|██████████▉ | 254/605 [00:54<01:13, 4.79it/s]
Analyzing ProxyRecord: 42%|██████████▉ | 255/605 [00:54<01:13, 4.78it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 42%|███████████ | 256/605 [00:54<01:13, 4.76it/s]
Analyzing ProxyRecord: 42%|███████████ | 257/605 [00:54<01:13, 4.75it/s]
Analyzing ProxyRecord: 43%|███████████ | 258/605 [00:54<01:12, 4.76it/s]
Analyzing ProxyRecord: 43%|███████████▏ | 259/605 [00:55<01:12, 4.78it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 43%|███████████▏ | 260/605 [00:55<01:12, 4.75it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 43%|███████████▏ | 261/605 [00:55<01:12, 4.74it/s]
Analyzing ProxyRecord: 43%|███████████▎ | 262/605 [00:55<01:12, 4.72it/s]
Analyzing ProxyRecord: 43%|███████████▎ | 263/605 [00:55<01:12, 4.74it/s]
Analyzing ProxyRecord: 44%|███████████▎ | 264/605 [00:56<01:11, 4.76it/s]
Analyzing ProxyRecord: 44%|███████████▍ | 265/605 [00:56<01:11, 4.78it/s]
Analyzing ProxyRecord: 44%|███████████▍ | 266/605 [00:56<01:10, 4.79it/s]
Analyzing ProxyRecord: 44%|███████████▍ | 267/605 [00:56<01:10, 4.77it/s]
Analyzing ProxyRecord: 44%|███████████▌ | 268/605 [00:57<01:10, 4.78it/s]
Analyzing ProxyRecord: 44%|███████████▌ | 269/605 [00:57<01:10, 4.79it/s]
Analyzing ProxyRecord: 45%|███████████▌ | 270/605 [00:57<01:10, 4.76it/s]
Analyzing ProxyRecord: 45%|███████████▋ | 271/605 [00:57<01:10, 4.74it/s]
Analyzing ProxyRecord: 45%|███████████▋ | 272/605 [00:57<01:09, 4.78it/s]
Analyzing ProxyRecord: 45%|███████████▋ | 273/605 [00:58<01:09, 4.77it/s]
Analyzing ProxyRecord: 45%|███████████▊ | 274/605 [00:58<01:09, 4.75it/s]
Analyzing ProxyRecord: 45%|███████████▊ | 275/605 [00:58<01:10, 4.69it/s]
Analyzing ProxyRecord: 46%|███████████▊ | 276/605 [00:58<01:09, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 46%|███████████▉ | 277/605 [00:58<01:09, 4.70it/s]
Analyzing ProxyRecord: 46%|███████████▉ | 278/605 [00:59<01:09, 4.74it/s]
Analyzing ProxyRecord: 46%|███████████▉ | 279/605 [00:59<01:08, 4.74it/s]
Analyzing ProxyRecord: 46%|████████████ | 280/605 [00:59<01:08, 4.74it/s]
Analyzing ProxyRecord: 46%|████████████ | 281/605 [00:59<01:08, 4.76it/s]
Analyzing ProxyRecord: 47%|████████████ | 282/605 [00:59<01:07, 4.80it/s]
Analyzing ProxyRecord: 47%|████████████▏ | 283/605 [01:00<01:07, 4.78it/s]
Analyzing ProxyRecord: 47%|████████████▏ | 284/605 [01:00<01:07, 4.75it/s]
Analyzing ProxyRecord: 47%|████████████▏ | 285/605 [01:00<01:07, 4.73it/s]
Analyzing ProxyRecord: 47%|████████████▎ | 286/605 [01:00<01:07, 4.76it/s]
Analyzing ProxyRecord: 47%|████████████▎ | 287/605 [01:01<01:06, 4.79it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 48%|████████████▍ | 288/605 [01:01<01:06, 4.79it/s]
Analyzing ProxyRecord: 48%|████████████▍ | 289/605 [01:01<01:06, 4.76it/s]
Analyzing ProxyRecord: 48%|████████████▍ | 290/605 [01:01<01:06, 4.75it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 48%|████████████▌ | 291/605 [01:01<01:06, 4.74it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 48%|████████████▌ | 292/605 [01:02<01:06, 4.71it/s]
Analyzing ProxyRecord: 48%|████████████▌ | 293/605 [01:02<01:05, 4.74it/s]
Analyzing ProxyRecord: 49%|████████████▋ | 294/605 [01:02<01:05, 4.73it/s]
Analyzing ProxyRecord: 49%|████████████▋ | 295/605 [01:02<01:05, 4.75it/s]
Analyzing ProxyRecord: 49%|████████████▋ | 296/605 [01:02<01:05, 4.75it/s]
Analyzing ProxyRecord: 49%|████████████▊ | 297/605 [01:03<01:04, 4.78it/s]
Analyzing ProxyRecord: 49%|████████████▊ | 298/605 [01:03<01:04, 4.77it/s]
Analyzing ProxyRecord: 49%|████████████▊ | 299/605 [01:03<01:04, 4.72it/s]
Analyzing ProxyRecord: 50%|████████████▉ | 300/605 [01:03<01:04, 4.73it/s]
Analyzing ProxyRecord: 50%|████████████▉ | 301/605 [01:03<01:04, 4.75it/s]
Analyzing ProxyRecord: 50%|████████████▉ | 302/605 [01:04<01:03, 4.77it/s]
Analyzing ProxyRecord: 50%|█████████████ | 303/605 [01:04<01:07, 4.45it/s]
Analyzing ProxyRecord: 50%|█████████████ | 304/605 [01:04<01:06, 4.52it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 50%|█████████████ | 305/605 [01:04<01:05, 4.57it/s]
Analyzing ProxyRecord: 51%|█████████████▏ | 306/605 [01:05<01:04, 4.63it/s]
Analyzing ProxyRecord: 51%|█████████████▏ | 307/605 [01:05<01:04, 4.65it/s]
Analyzing ProxyRecord: 51%|█████████████▏ | 308/605 [01:05<01:03, 4.67it/s]
Analyzing ProxyRecord: 51%|█████████████▎ | 309/605 [01:05<01:03, 4.70it/s]
Analyzing ProxyRecord: 51%|█████████████▎ | 310/605 [01:05<01:02, 4.72it/s]
Analyzing ProxyRecord: 51%|█████████████▎ | 311/605 [01:06<01:01, 4.77it/s]
Analyzing ProxyRecord: 52%|█████████████▍ | 312/605 [01:06<01:01, 4.79it/s]
Analyzing ProxyRecord: 52%|█████████████▍ | 313/605 [01:06<01:00, 4.80it/s]
Analyzing ProxyRecord: 52%|█████████████▍ | 314/605 [01:06<01:00, 4.79it/s]
Analyzing ProxyRecord: 52%|█████████████▌ | 315/605 [01:07<01:10, 4.12it/s]
Analyzing ProxyRecord: 52%|█████████████▌ | 316/605 [01:07<01:07, 4.31it/s]
Analyzing ProxyRecord: 52%|█████████████▌ | 317/605 [01:07<01:05, 4.40it/s]
Analyzing ProxyRecord: 53%|█████████████▋ | 318/605 [01:07<01:03, 4.49it/s]
Analyzing ProxyRecord: 53%|█████████████▋ | 319/605 [01:07<01:02, 4.56it/s]
Analyzing ProxyRecord: 53%|█████████████▊ | 320/605 [01:08<01:01, 4.63it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 53%|█████████████▊ | 321/605 [01:08<01:01, 4.64it/s]
Analyzing ProxyRecord: 53%|█████████████▊ | 322/605 [01:08<01:00, 4.68it/s]
Analyzing ProxyRecord: 53%|█████████████▉ | 323/605 [01:08<00:59, 4.71it/s]
Analyzing ProxyRecord: 54%|█████████████▉ | 324/605 [01:08<00:59, 4.69it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 54%|█████████████▉ | 325/605 [01:09<00:59, 4.68it/s]
Analyzing ProxyRecord: 54%|██████████████ | 326/605 [01:09<00:59, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 54%|██████████████ | 327/605 [01:09<00:58, 4.73it/s]
Analyzing ProxyRecord: 54%|██████████████ | 328/605 [01:09<00:58, 4.77it/s]
Analyzing ProxyRecord: 54%|██████████████▏ | 329/605 [01:10<00:58, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 55%|██████████████▏ | 330/605 [01:10<00:58, 4.73it/s]
Analyzing ProxyRecord: 55%|██████████████▏ | 331/605 [01:10<00:57, 4.75it/s]
Analyzing ProxyRecord: 55%|██████████████▎ | 332/605 [01:10<00:57, 4.74it/s]
Analyzing ProxyRecord: 55%|██████████████▎ | 333/605 [01:10<00:57, 4.75it/s]
Analyzing ProxyRecord: 55%|██████████████▎ | 334/605 [01:11<00:56, 4.78it/s]
Analyzing ProxyRecord: 55%|██████████████▍ | 335/605 [01:11<00:56, 4.79it/s]
Analyzing ProxyRecord: 56%|██████████████▍ | 336/605 [01:11<00:56, 4.74it/s]
Analyzing ProxyRecord: 56%|██████████████▍ | 337/605 [01:11<00:56, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 56%|██████████████▌ | 338/605 [01:11<00:56, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 56%|██████████████▌ | 339/605 [01:12<00:56, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 56%|██████████████▌ | 340/605 [01:12<00:56, 4.72it/s]
Analyzing ProxyRecord: 56%|██████████████▋ | 341/605 [01:12<00:55, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 57%|██████████████▋ | 342/605 [01:12<00:55, 4.72it/s]
Analyzing ProxyRecord: 57%|██████████████▋ | 343/605 [01:12<00:55, 4.76it/s]
Analyzing ProxyRecord: 57%|██████████████▊ | 344/605 [01:13<00:54, 4.75it/s]
Analyzing ProxyRecord: 57%|██████████████▊ | 345/605 [01:13<00:54, 4.77it/s]
Analyzing ProxyRecord: 57%|██████████████▊ | 346/605 [01:13<00:54, 4.75it/s]
Analyzing ProxyRecord: 57%|██████████████▉ | 347/605 [01:13<00:54, 4.75it/s]
Analyzing ProxyRecord: 58%|██████████████▉ | 348/605 [01:14<00:54, 4.73it/s]
Analyzing ProxyRecord: 58%|██████████████▉ | 349/605 [01:14<00:53, 4.77it/s]
Analyzing ProxyRecord: 58%|███████████████ | 350/605 [01:14<00:53, 4.78it/s]
Analyzing ProxyRecord: 58%|███████████████ | 351/605 [01:14<00:53, 4.77it/s]
Analyzing ProxyRecord: 58%|███████████████▏ | 352/605 [01:14<00:52, 4.79it/s]
Analyzing ProxyRecord: 58%|███████████████▏ | 353/605 [01:15<00:52, 4.79it/s]
Analyzing ProxyRecord: 59%|███████████████▏ | 354/605 [01:15<00:52, 4.78it/s]
Analyzing ProxyRecord: 59%|███████████████▎ | 355/605 [01:15<00:52, 4.77it/s]
Analyzing ProxyRecord: 59%|███████████████▎ | 356/605 [01:15<00:52, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 59%|███████████████▎ | 357/605 [01:15<00:52, 4.74it/s]
Analyzing ProxyRecord: 59%|███████████████▍ | 358/605 [01:16<00:51, 4.77it/s]
Analyzing ProxyRecord: 59%|███████████████▍ | 359/605 [01:16<00:51, 4.78it/s]
Analyzing ProxyRecord: 60%|███████████████▍ | 360/605 [01:16<00:51, 4.77it/s]
Analyzing ProxyRecord: 60%|███████████████▌ | 361/605 [01:16<00:51, 4.78it/s]
Analyzing ProxyRecord: 60%|███████████████▌ | 362/605 [01:16<00:51, 4.71it/s]
Analyzing ProxyRecord: 60%|███████████████▌ | 363/605 [01:17<00:51, 4.74it/s]
Analyzing ProxyRecord: 60%|███████████████▋ | 364/605 [01:17<00:50, 4.75it/s]
Analyzing ProxyRecord: 60%|███████████████▋ | 365/605 [01:17<00:50, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 60%|███████████████▋ | 366/605 [01:17<00:50, 4.76it/s]
Analyzing ProxyRecord: 61%|███████████████▊ | 367/605 [01:18<00:49, 4.77it/s]
Analyzing ProxyRecord: 61%|███████████████▊ | 368/605 [01:18<00:57, 4.13it/s]
Analyzing ProxyRecord: 61%|███████████████▊ | 369/605 [01:18<00:55, 4.29it/s]
Analyzing ProxyRecord: 61%|███████████████▉ | 370/605 [01:18<00:53, 4.41it/s]
Analyzing ProxyRecord: 61%|███████████████▉ | 371/605 [01:18<00:51, 4.53it/s]
Analyzing ProxyRecord: 61%|███████████████▉ | 372/605 [01:19<00:50, 4.62it/s]
Analyzing ProxyRecord: 62%|████████████████ | 373/605 [01:19<00:49, 4.67it/s]
Analyzing ProxyRecord: 62%|████████████████ | 374/605 [01:19<00:49, 4.71it/s]
Analyzing ProxyRecord: 62%|████████████████ | 375/605 [01:19<00:48, 4.72it/s]
Analyzing ProxyRecord: 62%|████████████████▏ | 376/605 [01:19<00:48, 4.75it/s]
Analyzing ProxyRecord: 62%|████████████████▏ | 377/605 [01:20<00:47, 4.79it/s]
Analyzing ProxyRecord: 62%|████████████████▏ | 378/605 [01:20<00:47, 4.79it/s]
Analyzing ProxyRecord: 63%|████████████████▎ | 379/605 [01:20<00:47, 4.79it/s]
Analyzing ProxyRecord: 63%|████████████████▎ | 380/605 [01:20<00:47, 4.77it/s]
Analyzing ProxyRecord: 63%|████████████████▎ | 381/605 [01:21<00:46, 4.80it/s]
Analyzing ProxyRecord: 63%|████████████████▍ | 382/605 [01:21<00:46, 4.80it/s]
Analyzing ProxyRecord: 63%|████████████████▍ | 383/605 [01:21<00:46, 4.77it/s]
Analyzing ProxyRecord: 63%|████████████████▌ | 384/605 [01:21<00:46, 4.75it/s]
Analyzing ProxyRecord: 64%|████████████████▌ | 385/605 [01:21<00:46, 4.76it/s]
Analyzing ProxyRecord: 64%|████████████████▌ | 386/605 [01:22<00:45, 4.77it/s]
Analyzing ProxyRecord: 64%|████████████████▋ | 387/605 [01:22<00:45, 4.77it/s]
Analyzing ProxyRecord: 64%|████████████████▋ | 388/605 [01:22<00:45, 4.75it/s]
Analyzing ProxyRecord: 64%|████████████████▋ | 389/605 [01:22<00:45, 4.76it/s]
Analyzing ProxyRecord: 64%|████████████████▊ | 390/605 [01:22<00:45, 4.75it/s]
Analyzing ProxyRecord: 65%|████████████████▊ | 391/605 [01:23<00:45, 4.74it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 65%|████████████████▊ | 392/605 [01:23<00:45, 4.72it/s]
Analyzing ProxyRecord: 65%|████████████████▉ | 393/605 [01:23<00:44, 4.73it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 65%|████████████████▉ | 394/605 [01:23<00:44, 4.70it/s]
Analyzing ProxyRecord: 65%|████████████████▉ | 395/605 [01:23<00:44, 4.73it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 65%|█████████████████ | 396/605 [01:24<00:44, 4.70it/s]
Analyzing ProxyRecord: 66%|█████████████████ | 397/605 [01:24<00:44, 4.72it/s]
Analyzing ProxyRecord: 66%|█████████████████ | 398/605 [01:24<00:43, 4.71it/s]
Analyzing ProxyRecord: 66%|█████████████████▏ | 399/605 [01:24<00:43, 4.73it/s]
Analyzing ProxyRecord: 66%|█████████████████▏ | 400/605 [01:25<00:43, 4.74it/s]
Analyzing ProxyRecord: 66%|█████████████████▏ | 401/605 [01:25<00:43, 4.73it/s]
Analyzing ProxyRecord: 66%|█████████████████▎ | 402/605 [01:25<00:42, 4.77it/s]
Analyzing ProxyRecord: 67%|█████████████████▎ | 403/605 [01:25<00:42, 4.78it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 67%|█████████████████▎ | 404/605 [01:25<00:48, 4.14it/s]
Analyzing ProxyRecord: 67%|█████████████████▍ | 405/605 [01:26<00:46, 4.30it/s]
Analyzing ProxyRecord: 67%|█████████████████▍ | 406/605 [01:26<00:44, 4.44it/s]
Analyzing ProxyRecord: 67%|█████████████████▍ | 407/605 [01:26<00:44, 4.45it/s]
Analyzing ProxyRecord: 67%|█████████████████▌ | 408/605 [01:26<00:44, 4.44it/s]
Analyzing ProxyRecord: 68%|█████████████████▌ | 409/605 [01:27<00:43, 4.52it/s]
Analyzing ProxyRecord: 68%|█████████████████▌ | 410/605 [01:27<00:42, 4.61it/s]
Analyzing ProxyRecord: 68%|█████████████████▋ | 411/605 [01:27<00:41, 4.66it/s]
Analyzing ProxyRecord: 68%|█████████████████▋ | 412/605 [01:27<00:41, 4.69it/s]
Analyzing ProxyRecord: 68%|█████████████████▋ | 413/605 [01:27<00:40, 4.69it/s]
Analyzing ProxyRecord: 68%|█████████████████▊ | 414/605 [01:28<00:40, 4.72it/s]
Analyzing ProxyRecord: 69%|█████████████████▊ | 415/605 [01:28<00:40, 4.71it/s]
Analyzing ProxyRecord: 69%|█████████████████▉ | 416/605 [01:28<00:40, 4.65it/s]
Analyzing ProxyRecord: 69%|█████████████████▉ | 417/605 [01:28<00:40, 4.70it/s]
Analyzing ProxyRecord: 69%|█████████████████▉ | 418/605 [01:28<00:39, 4.69it/s]
Analyzing ProxyRecord: 69%|██████████████████ | 419/605 [01:29<00:39, 4.72it/s]
Analyzing ProxyRecord: 69%|██████████████████ | 420/605 [01:29<00:39, 4.74it/s]
Analyzing ProxyRecord: 70%|██████████████████ | 421/605 [01:29<00:38, 4.73it/s]
Analyzing ProxyRecord: 70%|██████████████████▏ | 422/605 [01:29<00:38, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 70%|██████████████████▏ | 423/605 [01:30<00:38, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 70%|██████████████████▏ | 424/605 [01:30<00:38, 4.69it/s]
Analyzing ProxyRecord: 70%|██████████████████▎ | 425/605 [01:30<00:38, 4.71it/s]
Analyzing ProxyRecord: 70%|██████████████████▎ | 426/605 [01:30<00:37, 4.73it/s]
Analyzing ProxyRecord: 71%|██████████████████▎ | 427/605 [01:30<00:37, 4.70it/s]
Analyzing ProxyRecord: 71%|██████████████████▍ | 428/605 [01:31<00:37, 4.75it/s]
Analyzing ProxyRecord: 71%|██████████████████▍ | 429/605 [01:31<00:36, 4.76it/s]
Analyzing ProxyRecord: 71%|██████████████████▍ | 430/605 [01:31<00:36, 4.76it/s]
Analyzing ProxyRecord: 71%|██████████████████▌ | 431/605 [01:31<00:36, 4.74it/s]
Analyzing ProxyRecord: 71%|██████████████████▌ | 432/605 [01:31<00:36, 4.73it/s]
Analyzing ProxyRecord: 72%|██████████████████▌ | 433/605 [01:32<00:36, 4.76it/s]
Analyzing ProxyRecord: 72%|██████████████████▋ | 434/605 [01:32<00:41, 4.16it/s]
Analyzing ProxyRecord: 72%|██████████████████▋ | 435/605 [01:32<00:39, 4.28it/s]
Analyzing ProxyRecord: 72%|██████████████████▋ | 436/605 [01:32<00:38, 4.40it/s]
Analyzing ProxyRecord: 72%|██████████████████▊ | 437/605 [01:33<00:37, 4.49it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 72%|██████████████████▊ | 438/605 [01:33<00:36, 4.56it/s]
Analyzing ProxyRecord: 73%|██████████████████▊ | 439/605 [01:33<00:35, 4.61it/s]
Analyzing ProxyRecord: 73%|██████████████████▉ | 440/605 [01:33<00:35, 4.66it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 73%|██████████████████▉ | 441/605 [01:33<00:35, 4.67it/s]
Analyzing ProxyRecord: 73%|██████████████████▉ | 442/605 [01:34<00:34, 4.72it/s]
Analyzing ProxyRecord: 73%|███████████████████ | 443/605 [01:34<00:34, 4.72it/s]
Analyzing ProxyRecord: 73%|███████████████████ | 444/605 [01:34<00:34, 4.72it/s]
Analyzing ProxyRecord: 74%|███████████████████ | 445/605 [01:34<00:33, 4.72it/s]
Analyzing ProxyRecord: 74%|███████████████████▏ | 446/605 [01:34<00:33, 4.73it/s]
Analyzing ProxyRecord: 74%|███████████████████▏ | 447/605 [01:35<00:33, 4.72it/s]
Analyzing ProxyRecord: 74%|███████████████████▎ | 448/605 [01:35<00:33, 4.72it/s]
Analyzing ProxyRecord: 74%|███████████████████▎ | 449/605 [01:35<00:33, 4.72it/s]
Analyzing ProxyRecord: 74%|███████████████████▎ | 450/605 [01:35<00:32, 4.71it/s]
Analyzing ProxyRecord: 75%|███████████████████▍ | 451/605 [01:36<00:32, 4.71it/s]
Analyzing ProxyRecord: 75%|███████████████████▍ | 452/605 [01:36<00:32, 4.74it/s]
Analyzing ProxyRecord: 75%|███████████████████▍ | 453/605 [01:36<00:32, 4.71it/s]
Analyzing ProxyRecord: 75%|███████████████████▌ | 454/605 [01:36<00:32, 4.68it/s]
Analyzing ProxyRecord: 75%|███████████████████▌ | 455/605 [01:36<00:31, 4.69it/s]
Analyzing ProxyRecord: 75%|███████████████████▌ | 456/605 [01:37<00:31, 4.73it/s]
Analyzing ProxyRecord: 76%|███████████████████▋ | 457/605 [01:37<00:31, 4.72it/s]
Analyzing ProxyRecord: 76%|███████████████████▋ | 458/605 [01:37<00:30, 4.74it/s]
Analyzing ProxyRecord: 76%|███████████████████▋ | 459/605 [01:37<00:30, 4.72it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 76%|███████████████████▊ | 460/605 [01:37<00:30, 4.68it/s]
Analyzing ProxyRecord: 76%|███████████████████▊ | 461/605 [01:38<00:30, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 76%|███████████████████▊ | 462/605 [01:38<00:30, 4.67it/s]
Analyzing ProxyRecord: 77%|███████████████████▉ | 463/605 [01:38<00:30, 4.66it/s]
Analyzing ProxyRecord: 77%|███████████████████▉ | 464/605 [01:38<00:29, 4.70it/s]
Analyzing ProxyRecord: 77%|███████████████████▉ | 465/605 [01:39<00:29, 4.70it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 77%|████████████████████ | 466/605 [01:39<00:29, 4.68it/s]
Analyzing ProxyRecord: 77%|████████████████████ | 467/605 [01:39<00:29, 4.69it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 77%|████████████████████ | 468/605 [01:39<00:29, 4.69it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 78%|████████████████████▏ | 469/605 [01:39<00:29, 4.67it/s]
Analyzing ProxyRecord: 78%|████████████████████▏ | 470/605 [01:40<00:32, 4.10it/s]
Analyzing ProxyRecord: 78%|████████████████████▏ | 471/605 [01:40<00:31, 4.25it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 78%|████████████████████▎ | 472/605 [01:40<00:30, 4.35it/s]
Analyzing ProxyRecord: 78%|████████████████████▎ | 473/605 [01:40<00:29, 4.44it/s]
Analyzing ProxyRecord: 78%|████████████████████▎ | 474/605 [01:41<00:28, 4.54it/s]
Analyzing ProxyRecord: 79%|████████████████████▍ | 475/605 [01:41<00:28, 4.55it/s]
Analyzing ProxyRecord: 79%|████████████████████▍ | 476/605 [01:41<00:28, 4.55it/s]
Analyzing ProxyRecord: 79%|████████████████████▍ | 477/605 [01:41<00:27, 4.59it/s]
Analyzing ProxyRecord: 79%|████████████████████▌ | 478/605 [01:41<00:27, 4.63it/s]
Analyzing ProxyRecord: 79%|████████████████████▌ | 479/605 [01:42<00:26, 4.69it/s]
Analyzing ProxyRecord: 79%|████████████████████▋ | 480/605 [01:42<00:26, 4.70it/s]
Analyzing ProxyRecord: 80%|████████████████████▋ | 481/605 [01:42<00:26, 4.69it/s]
Analyzing ProxyRecord: 80%|████████████████████▋ | 482/605 [01:42<00:26, 4.70it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 80%|████████████████████▊ | 483/605 [01:42<00:25, 4.70it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 80%|████████████████████▊ | 484/605 [01:43<00:25, 4.67it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 80%|████████████████████▊ | 485/605 [01:43<00:25, 4.62it/s]
Analyzing ProxyRecord: 80%|████████████████████▉ | 486/605 [01:43<00:25, 4.65it/s]
Analyzing ProxyRecord: 80%|████████████████████▉ | 487/605 [01:43<00:25, 4.66it/s]
Analyzing ProxyRecord: 81%|████████████████████▉ | 488/605 [01:44<00:24, 4.68it/s]
Analyzing ProxyRecord: 81%|█████████████████████ | 489/605 [01:44<00:24, 4.69it/s]
Analyzing ProxyRecord: 81%|█████████████████████ | 490/605 [01:44<00:24, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 81%|█████████████████████ | 491/605 [01:44<00:24, 4.68it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 81%|█████████████████████▏ | 492/605 [01:44<00:24, 4.68it/s]
Analyzing ProxyRecord: 81%|█████████████████████▏ | 493/605 [01:45<00:23, 4.71it/s]
Analyzing ProxyRecord: 82%|█████████████████████▏ | 494/605 [01:45<00:23, 4.69it/s]
Analyzing ProxyRecord: 82%|█████████████████████▎ | 495/605 [01:45<00:23, 4.70it/s]
Analyzing ProxyRecord: 82%|█████████████████████▎ | 496/605 [01:45<00:23, 4.70it/s]
Analyzing ProxyRecord: 82%|█████████████████████▎ | 497/605 [01:46<00:26, 4.12it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 82%|█████████████████████▍ | 498/605 [01:46<00:25, 4.25it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 82%|█████████████████████▍ | 499/605 [01:46<00:24, 4.38it/s]
Analyzing ProxyRecord: 83%|█████████████████████▍ | 500/605 [01:46<00:23, 4.46it/s]
Analyzing ProxyRecord: 83%|█████████████████████▌ | 501/605 [01:46<00:23, 4.47it/s]
Analyzing ProxyRecord: 83%|█████████████████████▌ | 502/605 [01:47<00:22, 4.54it/s]
Analyzing ProxyRecord: 83%|█████████████████████▌ | 503/605 [01:47<00:22, 4.60it/s]
Analyzing ProxyRecord: 83%|█████████████████████▋ | 504/605 [01:47<00:21, 4.61it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 83%|█████████████████████▋ | 505/605 [01:47<00:21, 4.62it/s]
Analyzing ProxyRecord: 84%|█████████████████████▋ | 506/605 [01:47<00:21, 4.64it/s]
Analyzing ProxyRecord: 84%|█████████████████████▊ | 507/605 [01:48<00:20, 4.68it/s]
Analyzing ProxyRecord: 84%|█████████████████████▊ | 508/605 [01:48<00:20, 4.73it/s]
Analyzing ProxyRecord: 84%|█████████████████████▊ | 509/605 [01:48<00:20, 4.71it/s]
Analyzing ProxyRecord: 84%|█████████████████████▉ | 510/605 [01:48<00:20, 4.71it/s]
Analyzing ProxyRecord: 84%|█████████████████████▉ | 511/605 [01:49<00:19, 4.72it/s]
Analyzing ProxyRecord: 85%|██████████████████████ | 512/605 [01:49<00:19, 4.73it/s]
Analyzing ProxyRecord: 85%|██████████████████████ | 513/605 [01:49<00:19, 4.73it/s]
Analyzing ProxyRecord: 85%|██████████████████████ | 514/605 [01:49<00:19, 4.75it/s]
Analyzing ProxyRecord: 85%|██████████████████████▏ | 515/605 [01:49<00:18, 4.76it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 85%|██████████████████████▏ | 516/605 [01:50<00:18, 4.73it/s]
Analyzing ProxyRecord: 85%|██████████████████████▏ | 517/605 [01:50<00:18, 4.74it/s]
Analyzing ProxyRecord: 86%|██████████████████████▎ | 518/605 [01:50<00:18, 4.74it/s]
Analyzing ProxyRecord: 86%|██████████████████████▎ | 519/605 [01:50<00:18, 4.74it/s]
Analyzing ProxyRecord: 86%|██████████████████████▎ | 520/605 [01:51<00:20, 4.13it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 86%|██████████████████████▍ | 521/605 [01:51<00:19, 4.30it/s]
Analyzing ProxyRecord: 86%|██████████████████████▍ | 522/605 [01:51<00:18, 4.44it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 86%|██████████████████████▍ | 523/605 [01:51<00:18, 4.51it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 87%|██████████████████████▌ | 524/605 [01:51<00:17, 4.54it/s]
Analyzing ProxyRecord: 87%|██████████████████████▌ | 525/605 [01:52<00:17, 4.60it/s]
Analyzing ProxyRecord: 87%|██████████████████████▌ | 526/605 [01:52<00:17, 4.63it/s]
Analyzing ProxyRecord: 87%|██████████████████████▋ | 527/605 [01:52<00:16, 4.68it/s]
Analyzing ProxyRecord: 87%|██████████████████████▋ | 528/605 [01:52<00:16, 4.67it/s]
Analyzing ProxyRecord: 87%|██████████████████████▋ | 529/605 [01:52<00:16, 4.69it/s]
Analyzing ProxyRecord: 88%|██████████████████████▊ | 530/605 [01:53<00:15, 4.69it/s]
Analyzing ProxyRecord: 88%|██████████████████████▊ | 531/605 [01:53<00:15, 4.72it/s]
Analyzing ProxyRecord: 88%|██████████████████████▊ | 532/605 [01:53<00:15, 4.71it/s]
Analyzing ProxyRecord: 88%|██████████████████████▉ | 533/605 [01:53<00:15, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 88%|██████████████████████▉ | 534/605 [01:54<00:15, 4.70it/s]
Analyzing ProxyRecord: 88%|██████████████████████▉ | 535/605 [01:54<00:14, 4.68it/s]
Analyzing ProxyRecord: 89%|███████████████████████ | 536/605 [01:54<00:14, 4.68it/s]
Analyzing ProxyRecord: 89%|███████████████████████ | 537/605 [01:54<00:14, 4.66it/s]
Analyzing ProxyRecord: 89%|███████████████████████ | 538/605 [01:54<00:14, 4.67it/s]
Analyzing ProxyRecord: 89%|███████████████████████▏ | 539/605 [01:55<00:16, 4.11it/s]
Analyzing ProxyRecord: 89%|███████████████████████▏ | 540/605 [01:55<00:15, 4.26it/s]
Analyzing ProxyRecord: 89%|███████████████████████▏ | 541/605 [01:55<00:14, 4.38it/s]
Analyzing ProxyRecord: 90%|███████████████████████▎ | 542/605 [01:55<00:14, 4.47it/s]
Analyzing ProxyRecord: 90%|███████████████████████▎ | 543/605 [01:56<00:13, 4.53it/s]
Analyzing ProxyRecord: 90%|███████████████████████▍ | 544/605 [01:56<00:13, 4.60it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 90%|███████████████████████▍ | 545/605 [01:56<00:13, 4.61it/s]
Analyzing ProxyRecord: 90%|███████████████████████▍ | 546/605 [01:56<00:12, 4.65it/s]
Analyzing ProxyRecord: 90%|███████████████████████▌ | 547/605 [01:56<00:12, 4.68it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 91%|███████████████████████▌ | 548/605 [01:57<00:12, 4.69it/s]
Analyzing ProxyRecord: 91%|███████████████████████▌ | 549/605 [01:57<00:11, 4.71it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 91%|███████████████████████▋ | 550/605 [01:57<00:11, 4.72it/s]
Analyzing ProxyRecord: 91%|███████████████████████▋ | 551/605 [01:57<00:11, 4.69it/s]
Analyzing ProxyRecord: 91%|███████████████████████▋ | 552/605 [01:57<00:11, 4.70it/s]
Analyzing ProxyRecord: 91%|███████████████████████▊ | 553/605 [01:58<00:11, 4.72it/s]
Analyzing ProxyRecord: 92%|███████████████████████▊ | 554/605 [01:58<00:11, 4.61it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 92%|███████████████████████▊ | 555/605 [01:58<00:10, 4.56it/s]
Analyzing ProxyRecord: 92%|███████████████████████▉ | 556/605 [01:58<00:10, 4.50it/s]
Analyzing ProxyRecord: 92%|███████████████████████▉ | 557/605 [01:59<00:10, 4.42it/s]
Analyzing ProxyRecord: 92%|███████████████████████▉ | 558/605 [01:59<00:12, 3.86it/s]
Analyzing ProxyRecord: 92%|████████████████████████ | 559/605 [01:59<00:11, 4.00it/s]
Analyzing ProxyRecord: 93%|████████████████████████ | 560/605 [01:59<00:10, 4.12it/s]
Analyzing ProxyRecord: 93%|████████████████████████ | 561/605 [02:00<00:10, 4.23it/s]
Analyzing ProxyRecord: 93%|████████████████████████▏ | 562/605 [02:00<00:09, 4.35it/s]
Analyzing ProxyRecord: 93%|████████████████████████▏ | 563/605 [02:00<00:09, 4.44it/s]
Analyzing ProxyRecord: 93%|████████████████████████▏ | 564/605 [02:00<00:09, 4.51it/s]
Analyzing ProxyRecord: 93%|████████████████████████▎ | 565/605 [02:00<00:08, 4.57it/s]
Analyzing ProxyRecord: 94%|████████████████████████▎ | 566/605 [02:01<00:08, 4.62it/s]
Analyzing ProxyRecord: 94%|████████████████████████▎ | 567/605 [02:01<00:08, 4.64it/s]
Analyzing ProxyRecord: 94%|████████████████████████▍ | 568/605 [02:01<00:07, 4.66it/s]
Analyzing ProxyRecord: 94%|████████████████████████▍ | 569/605 [02:01<00:07, 4.68it/s]
Analyzing ProxyRecord: 94%|████████████████████████▍ | 570/605 [02:02<00:07, 4.66it/s]
Analyzing ProxyRecord: 94%|████████████████████████▌ | 571/605 [02:02<00:07, 4.69it/s]
Analyzing ProxyRecord: 95%|████████████████████████▌ | 572/605 [02:02<00:07, 4.66it/s]
Analyzing ProxyRecord: 95%|████████████████████████▌ | 573/605 [02:02<00:06, 4.64it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 95%|████████████████████████▋ | 574/605 [02:02<00:06, 4.66it/s]
Analyzing ProxyRecord: 95%|████████████████████████▋ | 575/605 [02:03<00:06, 4.65it/s]
Analyzing ProxyRecord: 95%|████████████████████████▊ | 576/605 [02:03<00:06, 4.68it/s]
Analyzing ProxyRecord: 95%|████████████████████████▊ | 577/605 [02:03<00:05, 4.68it/s]
Analyzing ProxyRecord: 96%|████████████████████████▊ | 578/605 [02:03<00:05, 4.68it/s]
Analyzing ProxyRecord: 96%|████████████████████████▉ | 579/605 [02:03<00:05, 4.72it/s]
Analyzing ProxyRecord: 96%|████████████████████████▉ | 580/605 [02:04<00:05, 4.72it/s]
Analyzing ProxyRecord: 96%|████████████████████████▉ | 581/605 [02:04<00:05, 4.71it/s]
Analyzing ProxyRecord: 96%|█████████████████████████ | 582/605 [02:04<00:05, 4.09it/s]
Analyzing ProxyRecord: 96%|█████████████████████████ | 583/605 [02:04<00:05, 4.26it/s]
Analyzing ProxyRecord: 97%|█████████████████████████ | 584/605 [02:05<00:04, 4.39it/s]
Analyzing ProxyRecord: 97%|█████████████████████████▏| 585/605 [02:05<00:04, 4.47it/s]
Analyzing ProxyRecord: 97%|█████████████████████████▏| 586/605 [02:05<00:04, 4.54it/s]
Analyzing ProxyRecord: 97%|█████████████████████████▏| 587/605 [02:05<00:03, 4.58it/s]
Analyzing ProxyRecord: 97%|█████████████████████████▎| 588/605 [02:05<00:03, 4.63it/s]
Analyzing ProxyRecord: 97%|█████████████████████████▎| 589/605 [02:06<00:03, 4.66it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▎| 590/605 [02:06<00:03, 4.67it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▍| 591/605 [02:06<00:02, 4.67it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▍| 592/605 [02:06<00:02, 4.68it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▍| 593/605 [02:07<00:02, 4.69it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▌| 594/605 [02:07<00:02, 4.71it/s]
Analyzing ProxyRecord: 98%|█████████████████████████▌| 595/605 [02:07<00:02, 4.70it/s]
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/lib/function_base.py:518: RuntimeWarning: Mean of empty slice.
avg = a.mean(axis, **keepdims_kw)
/Users/deborahkhider/opt/anaconda3/envs/paleopandas/lib/python3.10/site-packages/numpy/core/_methods.py:192: RuntimeWarning: invalid value encountered in scalar divide
ret = ret.dtype.type(ret / rcount)
Analyzing ProxyRecord: 99%|█████████████████████████▌| 596/605 [02:07<00:01, 4.71it/s]
Analyzing ProxyRecord: 99%|█████████████████████████▋| 597/605 [02:07<00:01, 4.71it/s]
Analyzing ProxyRecord: 99%|█████████████████████████▋| 598/605 [02:08<00:01, 4.15it/s]
Analyzing ProxyRecord: 99%|█████████████████████████▋| 599/605 [02:08<00:01, 4.27it/s]
Analyzing ProxyRecord: 99%|█████████████████████████▊| 600/605 [02:08<00:01, 4.37it/s]
Analyzing ProxyRecord: 99%|█████████████████████████▊| 601/605 [02:08<00:00, 4.48it/s]
Analyzing ProxyRecord: 100%|█████████████████████████▊| 602/605 [02:09<00:00, 4.56it/s]
Analyzing ProxyRecord: 100%|█████████████████████████▉| 603/605 [02:09<00:00, 4.61it/s]
Analyzing ProxyRecord: 100%|█████████████████████████▉| 604/605 [02:09<00:00, 4.62it/s]
Analyzing ProxyRecord: 100%|██████████████████████████| 605/605 [02:09<00:00, 4.66it/s]
Analyzing ProxyRecord: 100%|██████████████████████████| 605/605 [02:09<00:00, 4.67it/s]
Bootstrapping: 0%| | 0/201 [00:00<?, ?it/s]
Bootstrapping: 1%|▎ | 2/201 [00:00<00:11, 17.15it/s]
Bootstrapping: 2%|▋ | 4/201 [00:00<00:11, 16.58it/s]
Bootstrapping: 3%|█ | 6/201 [00:00<00:11, 16.95it/s]
Bootstrapping: 4%|█▍ | 8/201 [00:00<00:11, 17.13it/s]
Bootstrapping: 5%|█▋ | 10/201 [00:00<00:11, 17.19it/s]
Bootstrapping: 6%|██ | 12/201 [00:00<00:10, 17.28it/s]
Bootstrapping: 7%|██▍ | 14/201 [00:00<00:10, 17.14it/s]
Bootstrapping: 8%|██▊ | 16/201 [00:00<00:10, 17.14it/s]
Bootstrapping: 9%|███▏ | 18/201 [00:01<00:10, 17.14it/s]
Bootstrapping: 10%|███▍ | 20/201 [00:01<00:10, 17.23it/s]
Bootstrapping: 11%|███▊ | 22/201 [00:01<00:10, 17.16it/s]
Bootstrapping: 12%|████▏ | 24/201 [00:01<00:10, 17.25it/s]
Bootstrapping: 13%|████▌ | 26/201 [00:01<00:10, 17.06it/s]
Bootstrapping: 14%|████▉ | 28/201 [00:01<00:10, 17.13it/s]
Bootstrapping: 15%|█████▏ | 30/201 [00:01<00:10, 16.93it/s]
Bootstrapping: 16%|█████▌ | 32/201 [00:01<00:09, 16.95it/s]
Bootstrapping: 17%|█████▉ | 34/201 [00:01<00:10, 16.64it/s]
Bootstrapping: 18%|██████▎ | 36/201 [00:02<00:09, 16.72it/s]
Bootstrapping: 19%|██████▌ | 38/201 [00:02<00:09, 16.91it/s]
Bootstrapping: 20%|██████▉ | 40/201 [00:02<00:09, 17.01it/s]
Bootstrapping: 21%|███████▎ | 42/201 [00:02<00:09, 17.11it/s]
Bootstrapping: 22%|███████▋ | 44/201 [00:02<00:09, 17.13it/s]
Bootstrapping: 23%|████████ | 46/201 [00:02<00:09, 15.56it/s]
Bootstrapping: 24%|████████▎ | 48/201 [00:02<00:09, 15.51it/s]
Bootstrapping: 25%|████████▋ | 50/201 [00:02<00:09, 15.78it/s]
Bootstrapping: 26%|█████████ | 52/201 [00:03<00:09, 16.12it/s]
Bootstrapping: 27%|█████████▍ | 54/201 [00:03<00:08, 16.42it/s]
Bootstrapping: 28%|█████████▊ | 56/201 [00:03<00:08, 16.69it/s]
Bootstrapping: 29%|██████████ | 58/201 [00:03<00:08, 16.90it/s]
Bootstrapping: 30%|██████████▍ | 60/201 [00:03<00:08, 17.01it/s]
Bootstrapping: 31%|██████████▊ | 62/201 [00:03<00:08, 17.06it/s]
Bootstrapping: 32%|███████████▏ | 64/201 [00:03<00:08, 17.12it/s]
Bootstrapping: 33%|███████████▍ | 66/201 [00:03<00:07, 17.12it/s]
Bootstrapping: 34%|███████████▊ | 68/201 [00:04<00:07, 17.01it/s]
Bootstrapping: 35%|████████████▏ | 70/201 [00:04<00:07, 17.14it/s]
Bootstrapping: 36%|████████████▌ | 72/201 [00:04<00:07, 16.81it/s]
Bootstrapping: 37%|████████████▉ | 74/201 [00:04<00:07, 16.84it/s]
Bootstrapping: 38%|█████████████▏ | 76/201 [00:04<00:07, 16.98it/s]
Bootstrapping: 39%|█████████████▌ | 78/201 [00:04<00:07, 17.07it/s]
Bootstrapping: 40%|█████████████▉ | 80/201 [00:04<00:07, 17.14it/s]
Bootstrapping: 41%|██████████████▎ | 82/201 [00:04<00:07, 16.82it/s]
Bootstrapping: 42%|██████████████▋ | 84/201 [00:04<00:07, 16.66it/s]
Bootstrapping: 43%|██████████████▉ | 86/201 [00:05<00:06, 16.81it/s]
Bootstrapping: 44%|███████████████▎ | 88/201 [00:05<00:06, 16.86it/s]
Bootstrapping: 45%|███████████████▋ | 90/201 [00:05<00:06, 16.95it/s]
Bootstrapping: 46%|████████████████ | 92/201 [00:05<00:06, 17.01it/s]
Bootstrapping: 47%|████████████████▎ | 94/201 [00:05<00:06, 17.06it/s]
Bootstrapping: 48%|████████████████▋ | 96/201 [00:05<00:06, 17.07it/s]
Bootstrapping: 49%|█████████████████ | 98/201 [00:05<00:06, 17.10it/s]
Bootstrapping: 50%|████████████████▉ | 100/201 [00:05<00:05, 17.04it/s]
Bootstrapping: 51%|█████████████████▎ | 102/201 [00:06<00:05, 16.70it/s]
Bootstrapping: 52%|█████████████████▌ | 104/201 [00:06<00:05, 16.82it/s]
Bootstrapping: 53%|█████████████████▉ | 106/201 [00:06<00:05, 16.81it/s]
Bootstrapping: 54%|██████████████████▎ | 108/201 [00:06<00:05, 16.86it/s]
Bootstrapping: 55%|██████████████████▌ | 110/201 [00:06<00:05, 16.95it/s]
Bootstrapping: 56%|██████████████████▉ | 112/201 [00:06<00:05, 16.97it/s]
Bootstrapping: 57%|███████████████████▎ | 114/201 [00:06<00:05, 16.93it/s]
Bootstrapping: 58%|███████████████████▌ | 116/201 [00:06<00:05, 16.92it/s]
Bootstrapping: 59%|███████████████████▉ | 118/201 [00:06<00:04, 16.88it/s]
Bootstrapping: 60%|████████████████████▎ | 120/201 [00:07<00:04, 16.88it/s]
Bootstrapping: 61%|████████████████████▋ | 122/201 [00:07<00:04, 16.42it/s]
Bootstrapping: 62%|████████████████████▉ | 124/201 [00:07<00:04, 16.54it/s]
Bootstrapping: 63%|█████████████████████▎ | 126/201 [00:07<00:04, 16.68it/s]
Bootstrapping: 64%|█████████████████████▋ | 128/201 [00:07<00:04, 16.77it/s]
Bootstrapping: 65%|█████████████████████▉ | 130/201 [00:07<00:04, 16.80it/s]
Bootstrapping: 66%|██████████████████████▎ | 132/201 [00:07<00:04, 16.75it/s]
Bootstrapping: 67%|██████████████████████▋ | 134/201 [00:07<00:03, 16.77it/s]
Bootstrapping: 68%|███████████████████████ | 136/201 [00:08<00:03, 16.78it/s]
Bootstrapping: 69%|███████████████████████▎ | 138/201 [00:08<00:03, 16.70it/s]
Bootstrapping: 70%|███████████████████████▋ | 140/201 [00:08<00:03, 16.74it/s]
Bootstrapping: 71%|████████████████████████ | 142/201 [00:08<00:03, 16.73it/s]
Bootstrapping: 72%|████████████████████████▎ | 144/201 [00:08<00:03, 16.74it/s]
Bootstrapping: 73%|████████████████████████▋ | 146/201 [00:08<00:03, 16.46it/s]
Bootstrapping: 74%|█████████████████████████ | 148/201 [00:08<00:03, 16.49it/s]
Bootstrapping: 75%|█████████████████████████▎ | 150/201 [00:08<00:03, 16.49it/s]
Bootstrapping: 76%|█████████████████████████▋ | 152/201 [00:09<00:02, 16.51it/s]
Bootstrapping: 77%|██████████████████████████ | 154/201 [00:09<00:02, 16.60it/s]
Bootstrapping: 78%|██████████████████████████▍ | 156/201 [00:09<00:02, 16.68it/s]
Bootstrapping: 79%|██████████████████████████▋ | 158/201 [00:09<00:02, 16.70it/s]
Bootstrapping: 80%|███████████████████████████ | 160/201 [00:09<00:02, 16.72it/s]
Bootstrapping: 81%|███████████████████████████▍ | 162/201 [00:09<00:02, 16.45it/s]
Bootstrapping: 82%|███████████████████████████▋ | 164/201 [00:09<00:02, 16.54it/s]
Bootstrapping: 83%|████████████████████████████ | 166/201 [00:09<00:02, 16.65it/s]
Bootstrapping: 84%|████████████████████████████▍ | 168/201 [00:10<00:02, 16.35it/s]
Bootstrapping: 85%|████████████████████████████▊ | 170/201 [00:10<00:01, 16.49it/s]
Bootstrapping: 86%|█████████████████████████████ | 172/201 [00:10<00:01, 16.66it/s]
Bootstrapping: 87%|█████████████████████████████▍ | 174/201 [00:10<00:01, 16.77it/s]
Bootstrapping: 88%|█████████████████████████████▊ | 176/201 [00:10<00:01, 16.53it/s]
Bootstrapping: 89%|██████████████████████████████ | 178/201 [00:10<00:01, 16.37it/s]
Bootstrapping: 90%|██████████████████████████████▍ | 180/201 [00:10<00:01, 16.57it/s]
Bootstrapping: 91%|██████████████████████████████▊ | 182/201 [00:10<00:01, 16.73it/s]
Bootstrapping: 92%|███████████████████████████████ | 184/201 [00:10<00:01, 16.84it/s]
Bootstrapping: 93%|███████████████████████████████▍ | 186/201 [00:11<00:00, 16.91it/s]
Bootstrapping: 94%|███████████████████████████████▊ | 188/201 [00:11<00:00, 16.98it/s]
Bootstrapping: 95%|████████████████████████████████▏ | 190/201 [00:11<00:00, 16.79it/s]
Bootstrapping: 96%|████████████████████████████████▍ | 192/201 [00:11<00:00, 16.92it/s]
Bootstrapping: 97%|████████████████████████████████▊ | 194/201 [00:11<00:00, 17.07it/s]
Bootstrapping: 98%|█████████████████████████████████▏| 196/201 [00:11<00:00, 17.08it/s]
Bootstrapping: 99%|█████████████████████████████████▍| 198/201 [00:11<00:00, 17.08it/s]
Bootstrapping: 100%|█████████████████████████████████▊| 200/201 [00:11<00:00, 17.08it/s]
Bootstrapping: 100%|██████████████████████████████████| 201/201 [00:11<00:00, 16.81it/s]
fig, ax = pdb.plot_composite()
current_title = ax['var'].title.get_text().split(',',1)[-1]
ax['var'].set_title('Multi-Proxy, '+current_title)
Text(0.5, 1.0, 'Multi-Proxy, 605 records, bin_width=10')
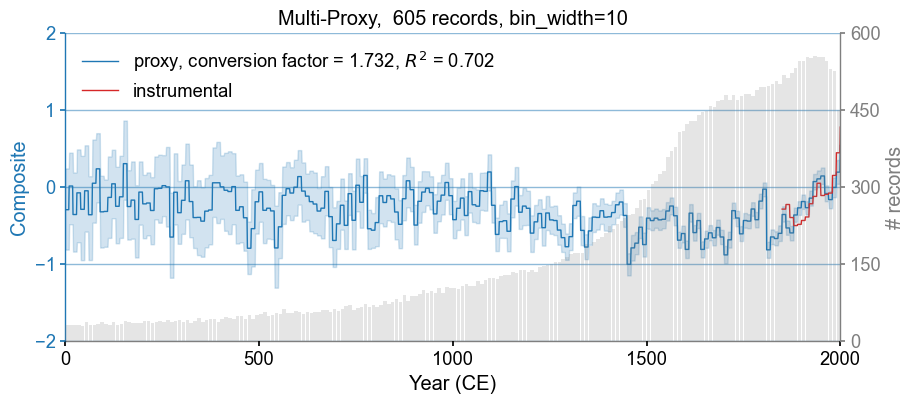
pdb.composite.keys()
dict_keys(['df_proxy', 'df_obs', 'proxy_comp', 'proxy_comp_time', 'proxy_comp_value', 'proxy_sq_low_time', 'proxy_sq_high_time', 'proxy_sq_low_value', 'proxy_sq_high_value', 'proxy_num', 'proxy_num_time', 'proxy_num_value', 'obs_comp', 'obs_comp_time', 'obs_comp_value', 'bin_width', 'intercept', 'slope', 'r2'])